Embarking on your Go (Golang) programming journey begins with setting up your development environment. This crucial initial step ensures you have the right tools and configurations for efficient Go program creation, compilation, and execution. A properly configured environment streamlines development and prevents common configuration errors.
This guide provides a comprehensive walkthrough of Go environment setup, covering essential concepts and offering a practical example. By the end, you'll have a functional Go environment and the skills to manage it effectively.
What You Will Learn:
- The importance of a well-structured Go environment.
- Core concepts: GOROOT, GOPATH, and Go modules.
- A step-by-step environment configuration guide.
- Best practices and common issues to avoid.
Core Concepts
Before the practical steps, let's understand key Go environment management concepts.
1. GOROOT
- Definition: GOROOT specifies the Go installation directory. It houses the Go standard library, compiler, and other essential tools.
-
Typical Location: Usually
/usr/local/go
on Unix-like systems andC:\Go
on Windows. -
Verification:
echo $GOROOT # Output (example): /usr/local/go
2. GOPATH
- Definition: GOPATH designates your workspace directory for Go projects and their dependencies. Go searches here for source code, binaries, and packages.
-
Default Location: Typically
$HOME/go
on Unix-like systems and%USERPROFILE%\go
on Windows. -
Verification:
echo $GOPATH # Output (example): /home/username/go
3. Go Modules
- Definition: Go modules are the standard dependency management system in Go (introduced in Go 1.11). They allow project-specific dependency management outside GOPATH.
-
Key Files:
go.mod
(defines the module and its dependencies) andgo.sum
(records the expected cryptographic checksums of dependencies). -
Initialization:
go mod init myproject
Practical Example: Setting Up a Go Project
Let's create a Go project to illustrate environment setup.
Step 1: Install Go
- Download: Download the appropriate installer from the official Go website.
- Installation: Follow the OS-specific installation instructions.
-
Verification:
echo $GOROOT # Output (example): /usr/local/go
Step 2: Configure Your Workspace
-
Create Workspace Directory:
echo $GOPATH # Output (example): /home/username/go
-
Initialize a Go Module:
go mod init myproject
Step 3: Create Your First Go Program
-
Create
main.go
:go version # Output (example): go version go1.20.1 linux/amd64
-
Run the Program:
mkdir -p ~/go/src/myproject cd ~/go/src/myproject
Step 4: Build and Install
-
Build:
go mod init myproject
-
Install:
package main import "fmt" func main() { fmt.Println("Hello, Go!") }
Best Practices
1. Utilize Go Modules
- Always use Go modules for dependency management. This is the modern and recommended approach.
- Example:
go run main.go # Output: Hello, Go!
2. Maintain Workspace Organization
- Keep your projects organized within GOPATH or use Go modules for external dependency management.
- Example Directory Structure:
go build
3. Avoid Common Issues
- Incorrect GOPATH: Ensure your GOPATH is correctly set. Incorrect settings can cause dependency resolution problems.
-
Ignoring
go.mod
: Always includego.mod
andgo.sum
in version control for reproducible builds.
Conclusion
Setting up your Go environment is fundamental. Understanding GOROOT, GOPATH, and Go modules enables a robust and efficient development environment. This guide provided a practical example and best practices to avoid common problems. Now, create your own Go project and explore the Go ecosystem!
Call to Action
Explore my website for more Golang tutorials and programming resources. Happy coding! ?
The above is the detailed content of Setting Up Your Go Environment. For more information, please follow other related articles on the PHP Chinese website!
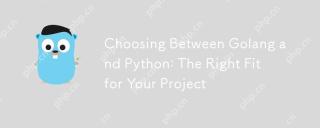
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
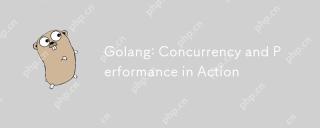
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
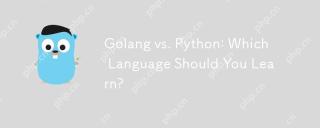
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
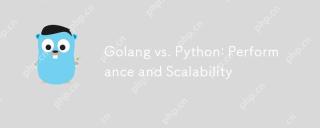
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
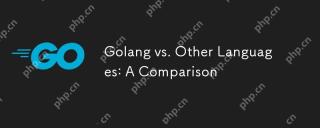
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
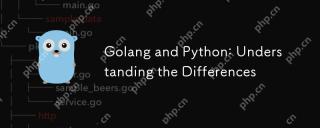
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
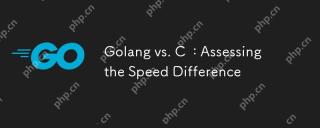
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
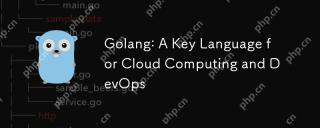
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
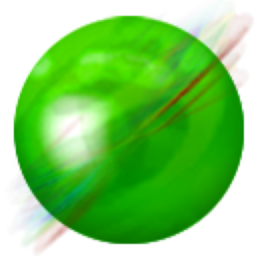
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
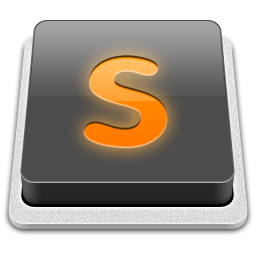
SublimeText3 Mac version
God-level code editing software (SublimeText3)
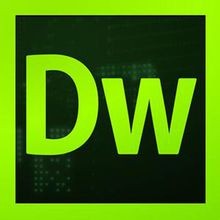
Dreamweaver CS6
Visual web development tools