Retrieve properties at runtime
This article introduces a general method for dynamically accessing and extracting attribute values of a class.
Use dedicated methods
Define a generic method that accepts type parameters:
public string GetDomainName<T>()
Internal method:
-
Use
typeof(T).GetCustomAttributes
to retrieve custom properties:var dnAttribute = typeof(T).GetCustomAttributes( typeof(DomainNameAttribute), true ).FirstOrDefault() as DomainNameAttribute;
-
If the attribute exists, return its value:
if (dnAttribute != null) { return dnAttribute.Name; }
-
Otherwise, return null:
return null;
Utility extension methods
For wider applicability, generalize this method to handle any attribute:
public static class AttributeExtensions { public static TValue GetAttributeValue<TAttribute, TValue>( this Type type, Func<TAttribute, TValue> valueSelector) where TAttribute : Attribute }
Internal extension method:
-
Retrieve custom attributes:
var att = type.GetCustomAttributes( typeof(TAttribute), true ).FirstOrDefault() as TAttribute;
-
If the attribute exists, use the provided
valueSelector
to extract the required value:if (att != null) { return valueSelector(att); }
-
Otherwise, return the default value of the type:
return default(TValue);
Usage examples
- Retrieve the
MyClass
attribute ofDomainName
:
string name = typeof(MyClass).GetDomainName<MyClass>();
- Retrieve any attribute value of
MyClass
using extension methods:
string name = typeof(MyClass) .GetAttributeValue<DomainNameAttribute, string>((DomainNameAttribute dna) => dna.Name);
The above is the detailed content of How Can I Dynamically Retrieve Attribute Values from a Class at Runtime?. For more information, please follow other related articles on the PHP Chinese website!
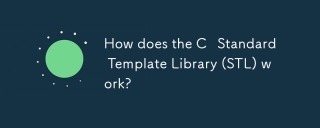
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
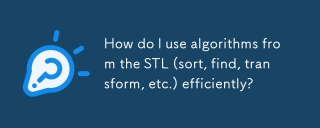
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
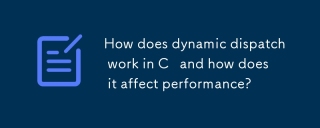
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
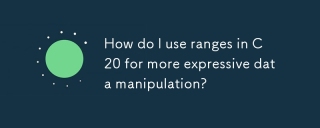
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
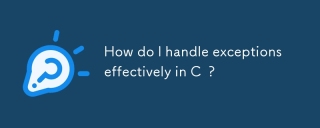
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
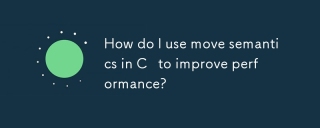
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
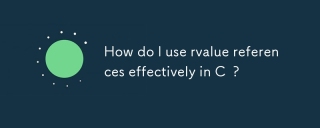
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
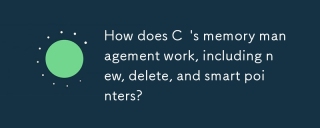
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
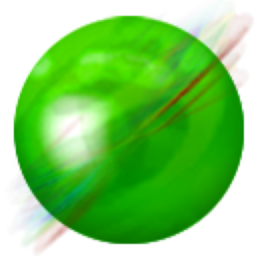
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
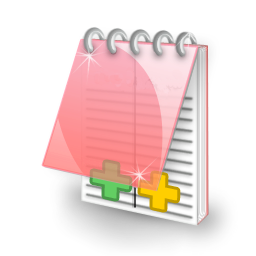
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
