Managing Processor Affinity for Threads and Tasks within .NET Applications
.NET applications offer methods to control which processors individual threads or tasks utilize. This fine-grained control allows for optimization of resource allocation.
Utilizing SetThreadAffinityMask
Traditionally, SetThreadAffinityMask
was employed to manage processor affinity at the thread level. This function, while functional, necessitates manual thread management, making it less convenient for many scenarios.
Leveraging Process
and ProcessThread
Objects
A more streamlined approach involves the ProcessorAffinity
property of the Process
and ProcessThread
objects. This property directly sets processor affinity for an entire process or individual threads within that process.
using System.Diagnostics; Process proc = Process.GetCurrentProcess(); long affinityMask = 0x000F; // Utilize processors 0-3 proc.ProcessorAffinity = (IntPtr)affinityMask; ProcessThread thread = proc.Threads[0]; affinityMask = 0x0002; // Utilize processor 1 thread.ProcessorAffinity = (IntPtr)affinityMask;
This example restricts the current process to the first four processors. The first thread is then further constrained to run only on the second processor.
Task Scheduler Preferences with IdealProcessor
While not guaranteeing specific processor assignment, the IdealProcessor
property of Thread
or Task
allows you to express a preference for a particular processor. The scheduler, however, retains ultimate control over thread-to-processor mapping.
Prioritizing Tasks for High CPU Utilization with TPL
The Task Parallel Library (TPL) lacks direct processor affinity settings for tasks. However, setting a task's Priority
property to ThreadPriority.Highest
enhances its execution priority, increasing its likelihood of consuming more CPU resources.
The above is the detailed content of How Can I Control Processor Affinity for Threads and Tasks in .NET?. For more information, please follow other related articles on the PHP Chinese website!
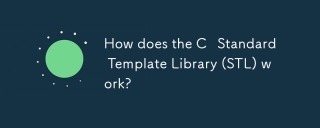
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
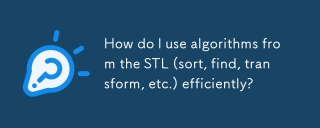
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
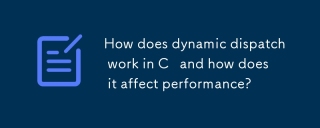
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
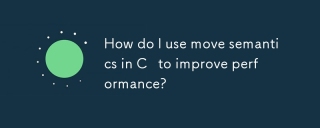
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
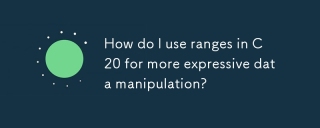
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
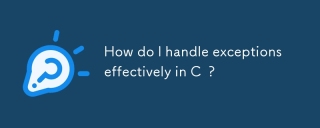
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
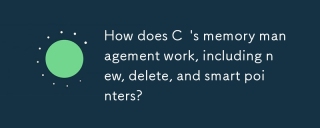
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
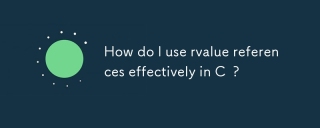
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
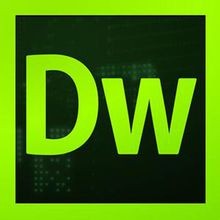
Dreamweaver CS6
Visual web development tools
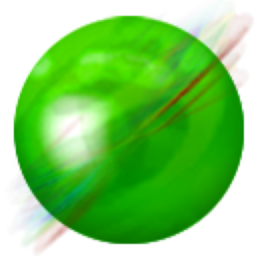
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
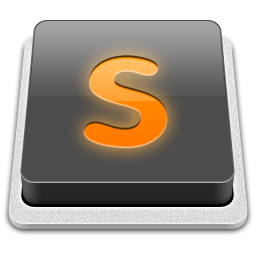
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
