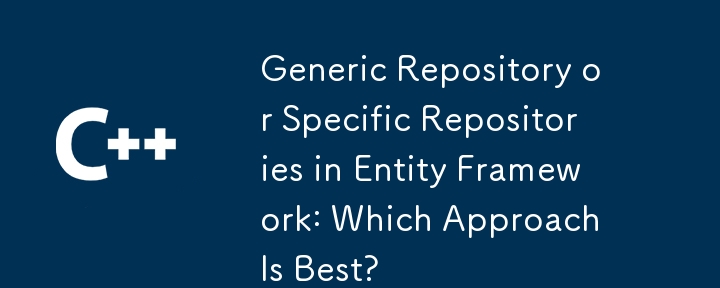
Entity Framework: General warehousing model or specific warehousing model?
In the database-first approach based on Entity Framework, a common question is: should you implement a common repository to manage context, or create separate repositories for each entity? While some advocate using generic repositories to encapsulate data access operations, this is generally considered an anti-pattern. Here’s why:
Advantages of specific warehousing:
-
Domain specificity: The warehousing should be consistent with the domain being modeled, and the domain itself is not generic. Different entities have different functions, and generic warehousing cannot fully express these characteristics.
-
Unique query mechanism: Query in a specific repository is unique for each entity, making a general approach inefficient. Generic repositories often result in complex predicate conditions that leak ORM-specific details to the service layer.
-
Composite Keys: Universal Repository cannot handle composite keys, which are common in many applications.
Disadvantages of universal warehousing:
-
Feature redundancy: EF already exposes a generic repository via DbSet, so implementing it would be redundant.
-
Complexity: In scenarios where you need to update specific fields or manage complex transactions, a universal repository can introduce unnecessary complexity.
Alternatives to general warehousing:
Instead of using a generic repository, consider the following:
-
Use ORM directly: If possible, use EF DbContext and DbSet directly in the calling code without an additional repository layer.
-
Concrete repository: If specific warehousing operations are required, create a specific repository that inherits from the simple general repository base class. This provides a level of abstraction without the drawbacks of generic repositories.
-
Specific query auxiliary methods: Define specific auxiliary methods in specific repositories to handle unique query scenarios that are not solved by the general repository.
Recommendation:
In most cases it is recommended to avoid using generic repositories and instead use EF directly or implement specific repositories as needed. This approach provides clear layering, eliminates unnecessary complexity, and ensures domain specificity.
The above is the detailed content of Generic Repository or Specific Repositories in Entity Framework: Which Approach Is Best?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn