.NET Asynchronous Programming: An in-depth discussion of Task.Start/Wait and Async/Await
.NET framework provides two asynchronous programming mechanisms: Task.Start/Wait and Async/Await. While the two have similarities, there are significant differences in behavior and impact.
Task.Start/Wait
The Task.Start/Wait method requires manually starting the task and then using the Wait() method to explicitly wait for its completion. This is a common pattern in synchronous programming and is suitable primarily for short-lived, non-blocking operations. The code using Task.Start/Wait is similar to:
public void MyMethod() { Task t = Task.Factory.StartNew(DoSomethingThatTakesTime); t.Wait(); UpdateLabelToSayItsComplete(); }
Create and start a new background task by calling Task.StartNew(). The Wait() method blocks the current thread until the task is completed, ensuring that subsequent code (such as updating labels) is only executed after the task is completed.
Async/Await
Async/Await (introduced in .NET 4.5) provides a more natural and efficient way to handle asynchronous operations without blocking the current thread. This approach leverages the concepts of collaborative multitasking and continuation. The code using Async/Await looks like this:
public async void MyMethod() { var result = Task.Factory.StartNew(DoSomethingThatTakesTime); await result; UpdateLabelToSayItsComplete(); }
The async keyword instructs the compiler to treat the method as an asynchronous method. When the await keyword is encountered, the current method is suspended, the asynchronous operation continues on the thread pool thread, and control is returned to the caller. When the awaited task completes, the continuation associated with await returns execution to the original method.
Key differences
The main difference between Task.Start/Wait and Async/Await is the way they handle threads. Task.Start/Wait creates and starts a background task that runs independently of the current thread. This can lead to too many threads if a large number of background tasks are created simultaneously.
Async/Await, on the other hand, does not create new threads. It yields the current thread to the thread pool and waits for the asynchronous operation to complete. Once completed, the continuation is executed on the thread pool thread that originally called the wait operation, ensuring correct thread semantics and reducing the risk of over-threading.
When to use which method
Task.Start/Wait is suitable for simple asynchronous operations that do not require additional updates or responses. It can also be used in situations where you need to block a thread intentionally, such as waiting for the user to confirm an operation before continuing.
Async/Await is ideal for long-running, user-interactive operations where the user interface must remain responsive and updated. It simplifies the processing of multiple asynchronous operations, avoids the complexity of manual task management, and ensures efficient thread utilization.
The above is the detailed content of Task.Start/Wait vs. Async/Await in .NET: When Should I Use Each Approach?. For more information, please follow other related articles on the PHP Chinese website!
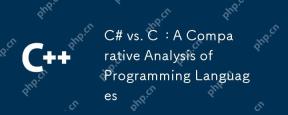
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
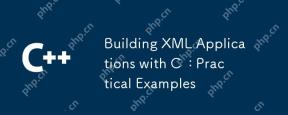
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
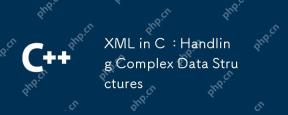
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
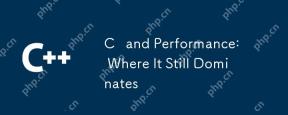
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
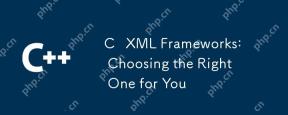
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
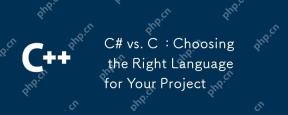
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
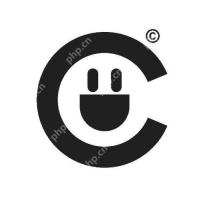
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
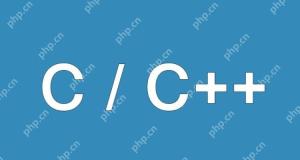
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
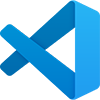
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
