Arrays and lists in C#: pass by reference or by value?
In C#, arrays and lists are passed by value. This means that when you pass these data structures as arguments to methods or functions, only copies of the references to them are passed.
Passed by value:
In .NET, arrays and lists are objects stored on the heap. When these objects are passed as arguments, references to their locations in memory are copied. This means that any changes made to the contents of an array or list are observable by the caller. However, if you reallocate the array or list itself (i.e. change the reference), these changes will not be detected by the caller.
Example:
void Foo(int[] data) { data[0] = 1; // 调用方可以看到此更改 } void Bar(int[] data) { data = new int[20]; // 调用方看不到此更改 }
Use ref for pass-by-reference:
If you need the caller to observe both content changes and reference reassignments, you can use the ref modifier. This will pass the reference by reference, ensuring that any modifications made to the reference itself are visible to the caller.
Example of using ref:
void Foo(ref int[] data) { data[0] = 1; // 调用方可以看到此更改 data = new int[20]; // 调用方也可以看到此更改 }
Thus, in C#, arrays and lists are passed by value by default. If you wish to pass the reference itself by reference, you can modify the method's signature to include the ref modifier.
The above is the detailed content of Are C# Arrays and Lists Passed by Value or by Reference?. For more information, please follow other related articles on the PHP Chinese website!
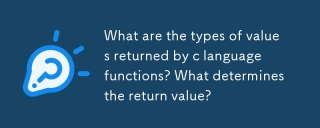
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
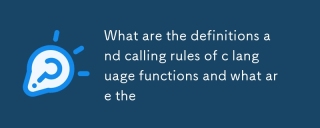
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
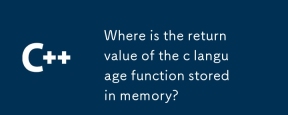
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
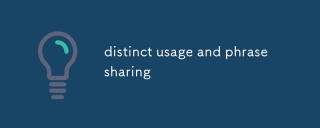
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
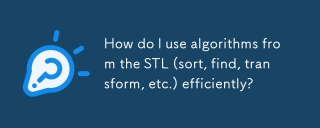
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
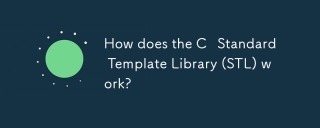
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
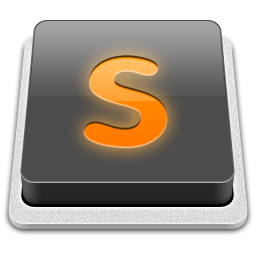
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
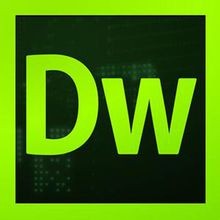
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
