


How to Efficiently Execute Stored Procedures and Retrieve Results in Classical ASP?
Classic ASP Stored Procedure Execution and Result Retrieval: Best Practices
Classic ASP developers often encounter challenges when working with SQL Server stored procedures, particularly regarding result retrieval. Empty responses or closed recordsets are common problems, even when the procedure should return data. This often stems from overlooking crucial SQL Server settings and inefficient data handling within the ASP code.
One frequent cause of empty results is neglecting to use SET NOCOUNT ON
in the stored procedure. By default, SQL Server returns the number of rows affected, which can interfere with the actual result set. SET NOCOUNT ON
ensures only the data is returned.
Another performance bottleneck is iterating through data using ADODB.Recordset
. This approach can be slow. A more efficient method is to retrieve the entire result set into an array for subsequent processing.
Optimized Approach for Classic ASP Stored Procedure Handling:
The following steps detail an optimized method for executing stored procedures and retrieving data in Classic ASP:
-
Instantiate
ADODB.Command
: Create anADODB.Command
object and establish a connection using a valid connection string or anADODB.Connection
object. -
Configure Command Properties: Set the
CommandText
property to the stored procedure name and theCommandType
property toadCmdStoredProc
. -
Manage Parameters: If the stored procedure accepts parameters, use the
Parameters
collection to add them, specifying data type and direction (e.g.,adParamInput
). -
Execute and Retrieve Data: Execute the command using the
Execute
method. Instead of directly processing the recordset, use theGetRows
method to efficiently load the entire result set into a two-dimensional array.
Revised Code Example:
Here's a refined code example demonstrating these improvements:
Const adParamInput = 1, adVarChar = 200 Dim conn_string, row, rows, ary_data conn_string = "PROVIDER=SQLOLEDB;DATA SOURCE=X;DATABASE=Y;UID=Z;PWD=W;" Set objCommandSec = CreateObject("ADODB.Command") With objCommandSec .ActiveConnection = conn_string .CommandType = adCmdStoredProc .CommandText = "usp_Targets_DataEntry_Display" .Parameters.Append .CreateParameter("@userinumber", adVarChar, adParamInput, 10, inumber) .Parameters.Append .CreateParameter("@group", adVarChar, adParamInput, 50, "ISM") .Parameters.Append .CreateParameter("@groupvalue", adVarChar, adParamInput, 50, ismID) .Parameters.Append .CreateParameter("@targettypeparam", adVarChar, adParamInput, 50, targetType) Set rs = .Execute() If Not rs.EOF Then ary_data = rs.GetRows() rs.Close() Set rs = Nothing End If End With Set objCommandSec = Nothing ' Process data from the array If IsArray(ary_data) Then rows = UBound(ary_data, 2) For row = 0 To rows Response.Write(ary_data(1, row) & "<br>") ' Example: Accessing the second column (index 1) Next Else Response.Write("No data returned") End If
This revised code efficiently retrieves data into an array, avoiding the performance overhead of recordset iteration, leading to faster and more reliable stored procedure execution in Classic ASP. Remember to handle potential errors appropriately in a production environment.
The above is the detailed content of How to Efficiently Execute Stored Procedures and Retrieve Results in Classical ASP?. For more information, please follow other related articles on the PHP Chinese website!
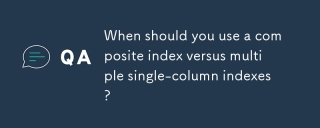
In database optimization, indexing strategies should be selected according to query requirements: 1. When the query involves multiple columns and the order of conditions is fixed, use composite indexes; 2. When the query involves multiple columns but the order of conditions is not fixed, use multiple single-column indexes. Composite indexes are suitable for optimizing multi-column queries, while single-column indexes are suitable for single-column queries.
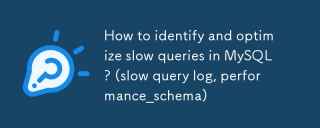
To optimize MySQL slow query, slowquerylog and performance_schema need to be used: 1. Enable slowquerylog and set thresholds to record slow query; 2. Use performance_schema to analyze query execution details, find out performance bottlenecks and optimize.
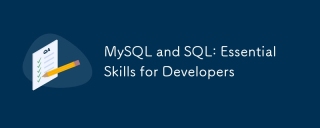
MySQL and SQL are essential skills for developers. 1.MySQL is an open source relational database management system, and SQL is the standard language used to manage and operate databases. 2.MySQL supports multiple storage engines through efficient data storage and retrieval functions, and SQL completes complex data operations through simple statements. 3. Examples of usage include basic queries and advanced queries, such as filtering and sorting by condition. 4. Common errors include syntax errors and performance issues, which can be optimized by checking SQL statements and using EXPLAIN commands. 5. Performance optimization techniques include using indexes, avoiding full table scanning, optimizing JOIN operations and improving code readability.
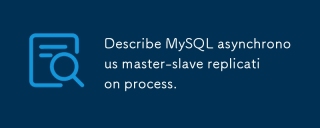
MySQL asynchronous master-slave replication enables data synchronization through binlog, improving read performance and high availability. 1) The master server record changes to binlog; 2) The slave server reads binlog through I/O threads; 3) The server SQL thread applies binlog to synchronize data.
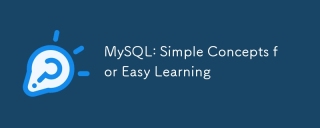
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
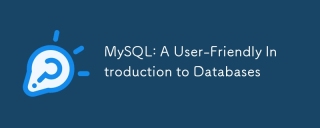
The installation and basic operations of MySQL include: 1. Download and install MySQL, set the root user password; 2. Use SQL commands to create databases and tables, such as CREATEDATABASE and CREATETABLE; 3. Execute CRUD operations, use INSERT, SELECT, UPDATE, DELETE commands; 4. Create indexes and stored procedures to optimize performance and implement complex logic. With these steps, you can build and manage MySQL databases from scratch.
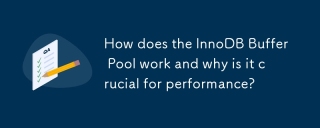
InnoDBBufferPool improves the performance of MySQL databases by loading data and index pages into memory. 1) The data page is loaded into the BufferPool to reduce disk I/O. 2) Dirty pages are marked and refreshed to disk regularly. 3) LRU algorithm management data page elimination. 4) The read-out mechanism loads the possible data pages in advance.
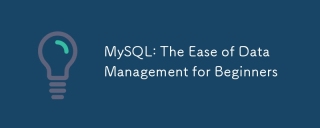
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
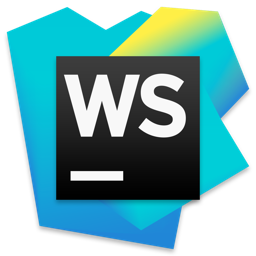
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.