Determine negotiated TLS version
When initiating a network handshake over TLS, it is often valuable for debugging or logging purposes to determine the specific TLS version that was agreed upon. This article explores ways to retrieve this information to suit a variety of scenarios.
Use .NET Reflection API
For .NET applications running on Framework 4.7 or higher, the following method leverages reflection to access the underlying TlsStream and extract the negotiated TLS protocol:
using System.Net; using System.Reflection; using System.Security.Authentication; // 获取与网络请求关联的TLS流 using (var requestStream = request.GetRequestStream()) { // 利用反射访问TLS流的属性 var tlsStream = requestStream.GetType() .GetProperty("Connection", BindingFlags.Instance | BindingFlags.NonPublic) .GetValue(requestStream); var tlsState = tlsStream.GetType() .GetProperty("NetworkStream", BindingFlags.Instance | BindingFlags.NonPublic) .GetValue(tlsStream); var sslProtocol = (SslProtocols)tlsState.GetType() .GetProperty("SslProtocol", BindingFlags.Instance | BindingFlags.NonPublic) .GetValue(tlsState); // 处理协商的TLS协议(例如,日志记录或显示) }
Use server certificate verification callback
Another approach involves using ServerCertificateValidationCallback, which is called when a TLS connection is established. Here's how this method is integrated:
using System.Net; using System.Security.Authentication; using System.Security.Cryptography.X509Certificates; // 设置ServerCertificateValidationCallback ServicePointManager.ServerCertificateValidationCallback += (sender, certificate, chain, errors) => { // 获取TLS协议版本 var sslProtocol = ((SslStream)sender).SslProtocol; // 处理协商的TLS协议(例如,日志记录或显示) return true; // 回调也可以用于证书验证,此处作为一个示例显示。 };
Use .NET Security DLL
Finally, an advanced technique involves using the QueryContextAttributesW method in secur32.dll to access the security context attributes. This method can provide more detailed information about the established secure connection.
using System; using System.Runtime.InteropServices; [DllImport("secur32.dll", CharSet = CharSet.Auto, ExactSpelling = true, SetLastError = false)] private static extern int QueryContextAttributesW( IntPtr contextHandle, ContextAttribute attribute, ref SecPkgContext_ConnectionInfo connectionInfo); public enum ContextAttribute { // 获取TLS协议版本 SecPkgContext_ConnectionInfo = 0x9 } public struct SecPkgContext_ConnectionInfo { public SchProtocols dwProtocol; // 其他属性也可以用于获取有关密码和哈希算法的信息 }
Note: This method requires access to non-public properties and fields, making it a less direct method.
By implementing these technologies, developers can retrieve negotiated TLS versions for HttpWebRequest and TcpClient connections, allowing them to capture valuable information for debugging and logging.
The above is the detailed content of How to Determine the Negotiated TLS Version in .NET Applications?. For more information, please follow other related articles on the PHP Chinese website!
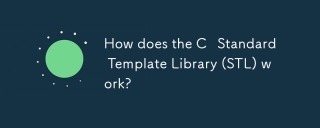
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
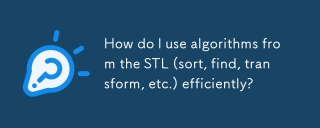
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
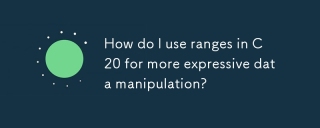
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
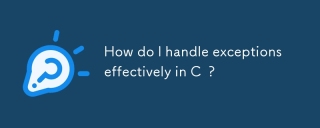
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
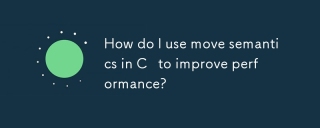
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
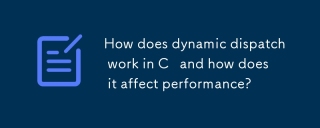
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
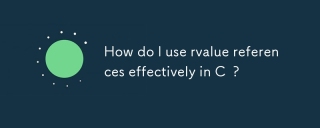
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
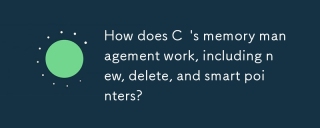
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
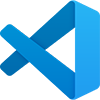
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
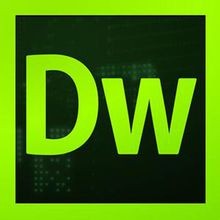
Dreamweaver CS6
Visual web development tools
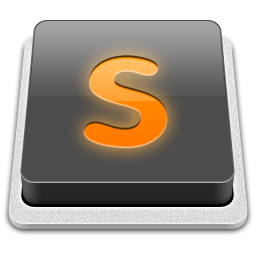
SublimeText3 Mac version
God-level code editing software (SublimeText3)