Managing state is a critical aspect of building scalable and performant React applications. Over the years, state management solutions have evolved significantly, providing developers with powerful tools to handle both local and global state efficiently. In 2025, the React ecosystem offers a plethora of options, from classic libraries like Redux to modern approaches like Zustand and Jotai. This article explores the current state of state management, compares popular tools, and provides practical guidance on choosing the right solution for your project.
Why State Management Matters
State management is essential for:
- Maintaining App Consistency: Ensures data flow and UI updates are synchronized.
- Scalability: Makes complex apps easier to manage.
- Collaboration: Simplifies teamwork by standardizing data handling.
Without proper state management, applications can become unmaintainable, leading to bugs, poor performance, and a negative user experience.
Popular State Management Solutions in 2025
1. Redux
Redux remains a popular choice for managing global state in large applications. Its unidirectional data flow and middleware ecosystem (like Redux Thunk and Redux Saga) make it a powerful tool for handling complex state logic.
Pros:
- Predictable state updates.
- Strong community support and ecosystem.
Cons:
- Verbose boilerplate code.
- Steeper learning curve for beginners.
Example:
import { createSlice, configureStore } from '@reduxjs/toolkit'; const counterSlice = createSlice({ name: 'counter', initialState: { value: 0 }, reducers: { increment: (state) => { state.value += 1; }, decrement: (state) => { state.value -= 1; }, }, }); export const { increment, decrement } = counterSlice.actions; const store = configureStore({ reducer: { counter: counterSlice.reducer } });
2. Context API
The Context API is built into React and is ideal for managing small to medium-sized global state.
Pros:
- Simple and lightweight.
- No external libraries required.
Cons:
- Can lead to performance issues with frequent re-renders.
Example:
import React, { createContext, useContext, useState } from 'react'; const CounterContext = createContext(); export function CounterProvider({ children }) { const [count, setCount] = useState(0); return ( <countercontext.provider value="{{" count setcount> {children} </countercontext.provider> ); } export function useCounter() { return useContext(CounterContext); }
3. Zustand
Zustand is a lightweight state management library that uses a minimalistic API to handle state with ease.
Pros:
- Simple API with minimal boilerplate.
- Excellent performance.
Cons:
- Smaller community compared to Redux.
Example:
import create from 'zustand'; const useStore = create((set) => ({ count: 0, increment: () => set((state) => ({ count: state.count + 1 })), decrement: () => set((state) => ({ count: state.count - 1 })), })); function Counter() { const { count, increment, decrement } = useStore(); return ( <div> <button onclick="{decrement}">-</button> <span>{count}</span> <button onclick="{increment}">+</button> </div> ); }
4. Jotai
Jotai is an atomic state management library inspired by Recoil. It offers flexible and composable state handling.
Pros:
- Focuses on atomic states for better modularity.
- Lightweight and performant.
Cons:
- Smaller ecosystem compared to Redux.
Example:
import { createSlice, configureStore } from '@reduxjs/toolkit'; const counterSlice = createSlice({ name: 'counter', initialState: { value: 0 }, reducers: { increment: (state) => { state.value += 1; }, decrement: (state) => { state.value -= 1; }, }, }); export const { increment, decrement } = counterSlice.actions; const store = configureStore({ reducer: { counter: counterSlice.reducer } });
5. React Query (TanStack Query)
React Query excels at managing server state, including caching, synchronization, and background updates.
Pros:
- Optimized for server state management.
- Simplifies API integration.
Cons:
- Not suitable for local state.
Example:
import React, { createContext, useContext, useState } from 'react'; const CounterContext = createContext(); export function CounterProvider({ children }) { const [count, setCount] = useState(0); return ( <countercontext.provider value="{{" count setcount> {children} </countercontext.provider> ); } export function useCounter() { return useContext(CounterContext); }
Choosing the Right Solution
When selecting a state management solution, consider the following:
- Application Size: For small apps, Context API or Zustand may suffice. For larger apps, consider Redux or Jotai.
- State Type: Use React Query for server state and Redux or Zustand for local/global state.
- Performance Needs: Evaluate the performance trade-offs, especially with frequent state updates.
- Developer Experience: Choose a tool that aligns with your team’s expertise and project requirements.
Conclusion
State management in React has come a long way, offering developers a wide range of tools to tackle both simple and complex scenarios. As of 2025, solutions like Redux, Zustand, Jotai, and React Query each have their place, depending on the needs of your project. By understanding the strengths and limitations of each, you can make informed decisions and build scalable, maintainable applications.
Are you using any of these state management solutions? Share your experiences and tips in the comments below!
The above is the detailed content of State Management in React Exploring Modern Solutions. For more information, please follow other related articles on the PHP Chinese website!
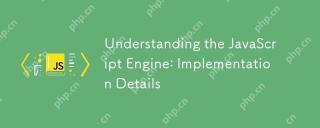
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
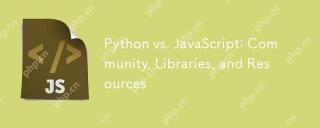
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
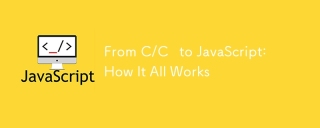
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
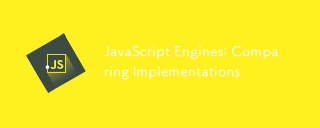
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
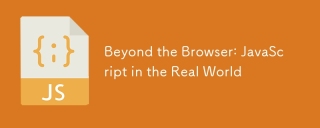
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
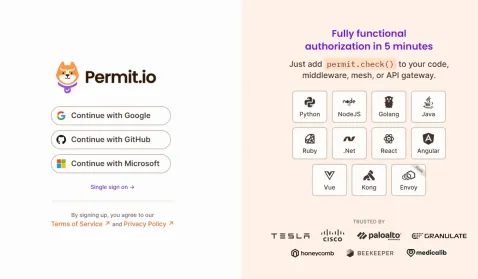
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
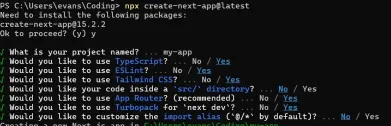
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
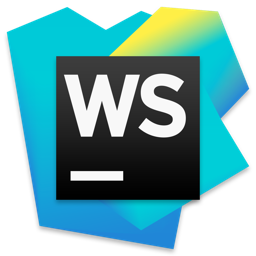
WebStorm Mac version
Useful JavaScript development tools
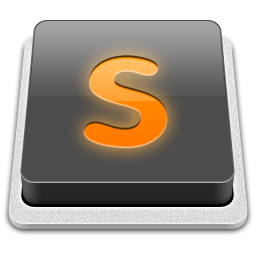
SublimeText3 Mac version
God-level code editing software (SublimeText3)