Unit Testing Web Services: Handling HttpContext.Current.Session
Unit testing web services often requires managing HttpContext.Current.Session
to avoid null reference exceptions. Directly accessing the session without proper setup will fail. While mocking HttpContext
using SimpleWorkerRequest
is common, setting session values using HttpContext.Current.Session["key"] = "value"
often results in errors because the session isn't initialized.
The solution involves accurately simulating the session within the unit test. This can be achieved by creating a HttpContext
with a custom session container.
Method 1: Manual HttpContext and Session Creation
This method directly constructs the HttpContext
and its session:
public static HttpContext FakeHttpContext() { var httpRequest = new HttpRequest("", "http://example.com/", ""); var stringWriter = new StringWriter(); var httpResponse = new HttpResponse(stringWriter); var httpContext = new HttpContext(httpRequest, httpResponse); var sessionContainer = new HttpSessionStateContainer("id", new SessionStateItemCollection(), new HttpStaticObjectsCollection(), 10, true, HttpCookieMode.AutoDetect, SessionStateMode.InProc, false); httpContext.Items["AspSession"] = typeof(HttpSessionState).GetConstructor( BindingFlags.NonPublic | BindingFlags.Instance, null, CallingConventions.Standard, new[] { typeof(HttpSessionStateContainer) }, null) .Invoke(new object[] { sessionContainer }); return httpContext; }
Method 2: Using SessionStateUtility
A more concise approach utilizes the SessionStateUtility
class:
SessionStateUtility.AddHttpSessionStateToContext(httpContext, sessionContainer);
This simplifies the process of attaching the session container to the HttpContext
. Remember to include the necessary using statements for both methods.
By employing either of these methods, you effectively simulate a functional HttpContext
with an initialized session, allowing you to set and retrieve session values within your unit tests. This ensures reliable and accurate testing of your web service logic.
The above is the detailed content of How to Initialize HttpContext.Current.Session for Unit Testing?. For more information, please follow other related articles on the PHP Chinese website!
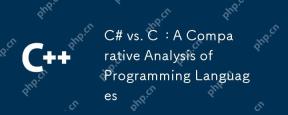
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
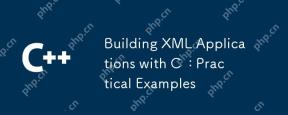
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
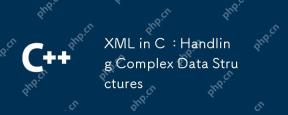
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
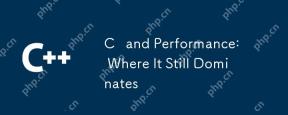
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
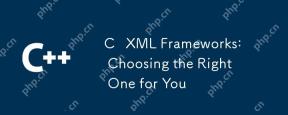
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
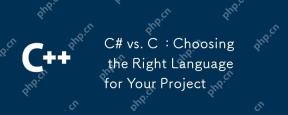
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
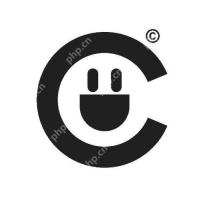
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
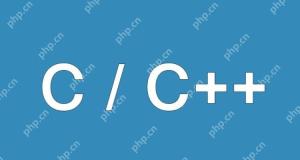
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
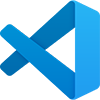
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
