Simplifying String Repetition with String Builders
The task of inserting indentations before a string can be simplified by repeating a string a specified number of times. To efficiently achieve this, consider utilizing the String.Repeat method.
String.Repeat
The String.Repeat method takes a single string parameter and an integer representing the desired number of repetitions. It returns a new string with the original string concatenated upon itself the specified number of times.
Usage
Here's an example of using String.Repeat to create a variable number of indentations:
// Define the indentation string string indent = "---"; // Repeat the indentation string based on an item's depth int depth = 3; string indentation = indent.Repeat(depth); // Display the indented string Console.WriteLine(indentation + "Example String");
In this example, the indentation variable is assigned a string with three dashes, and the indented string is displayed with three levels of indentation.
String Constructor
An alternative approach for repeating a single character is using the String constructor that takes a character and the number of repetitions.
// Repeat a dash five times char dash = '-'; int repetitions = 5; string result = new String(dash, repetitions);
This approach is particularly convenient when repeating a single non-alphanumeric character, resulting in a string of a specified length filled with the character.
The above is the detailed content of How Can I Efficiently Repeat a String Multiple Times in C#?. For more information, please follow other related articles on the PHP Chinese website!
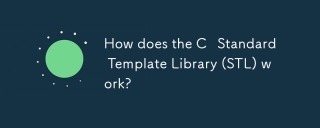
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
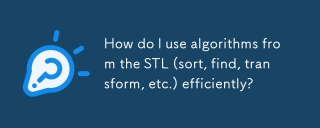
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
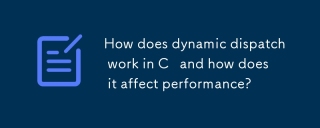
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
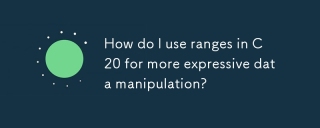
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
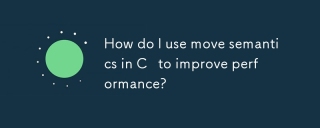
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
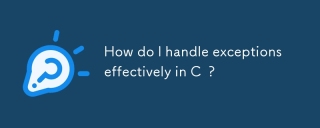
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
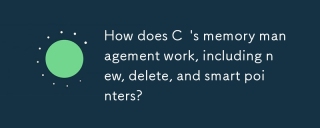
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
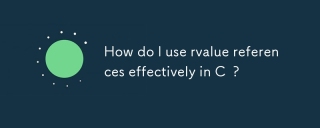
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
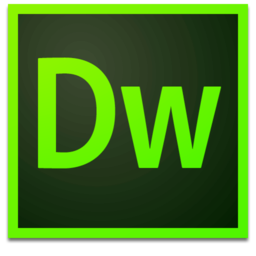
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
