


Referencing Class Fields in C#
In C#, passing a parameter by reference allows for direct modification of the original variable. However, when assigning a reference parameter to a class field, the expected behavior may not occur.
Consider the following code:
public class X { public X() { string example = "X"; new Y(ref example); new Z(ref example); System.Diagnostics.Debug.WriteLine(example); } } public class Y { public Y(ref string example) { example += " (Updated By Y)"; } } public class Z { private string _Example; public Z(ref string example) { this._Example = example; this._Example += " (Updated By Z)"; } } var x = new X();
Upon execution, the output is "X (Updated By Y)", not "X (Updated By Y) (Updated By Z)" as anticipated.
This is because assigning a reference parameter to a class field breaks the reference. To maintain the reference, a solution is to use a getter and setter:
sealed class Ref<t> { private readonly Func<t> getter; private readonly Action<t> setter; public Ref(Func<t> getter, Action<t> setter) { this.getter = getter; this.setter = setter; } public T Value { get { return getter(); } set { setter(value); } } } Ref<int> x; void M() { int y = 123; x = new Ref<int>(() => y, z => { y = z; }); x.Value = 456; Console.WriteLine(y); // 456 -- setting x.Value changes y. }</int></int></t></t></t></t></t>
In this example, 'x' is an object that can get and set the value of 'y', maintaining the reference between them. Note that while ref locals and ref-returning methods are supported in the CLR, they are not yet available in C#.
The above is the detailed content of Why Doesn't Assigning a Reference Parameter to a Class Field Maintain the Reference in C#?. For more information, please follow other related articles on the PHP Chinese website!
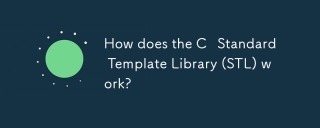
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
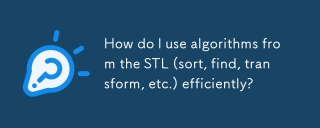
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
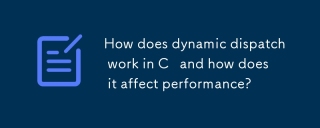
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
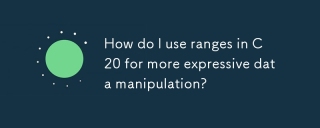
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
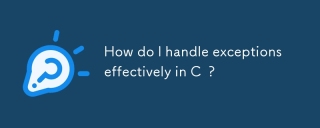
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
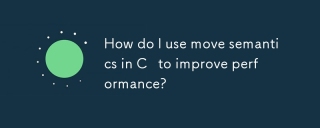
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
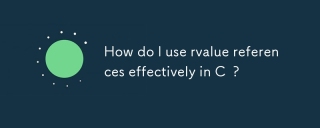
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
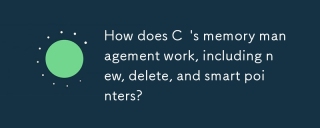
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
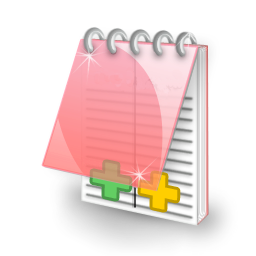
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
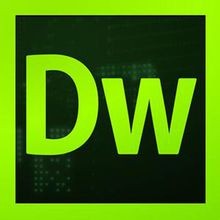
Dreamweaver CS6
Visual web development tools
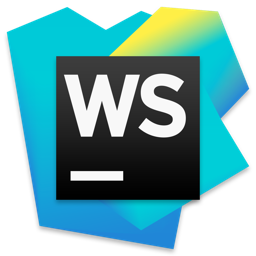
WebStorm Mac version
Useful JavaScript development tools
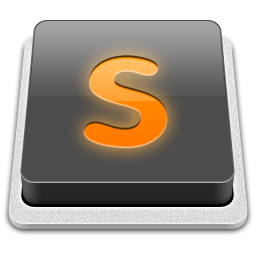
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
