Start at the Basics
With the advent of AI and some tech-ed influencers, there seem to be alot of skipping of the essentials before using a framework in Javascript land. Understanding core JavaScript concepts is crucial, its like learning to walk before running. When I got this new job and had to get decent at understanding Vue, I took time to review these JavaScript to have an effective approach to Vue 3 development, I understand aand can use React ... but it isn't NOT my favorite framework, this is another discussion. Here's why these fundamentals matter :
Variables and Data Types
- Why it matters: Vue 3's reactivity system relies heavily on proper variable declarations.
- The composition API requires an understanding of const for refs and reactive objects.
- Type awareness helps with Vue3's template rendering and prop validation.
const count = ref(0) const user = reactive({ name: 'John', age: 30 })
Template Literals
- Why it matters: This is essential for Vue3 template expressions and string interpolation.
- It is extensively used in computed properties and methods.
- Template literals can be helpful for dynamic component templates and prop values.
const greeting = computed(() => `Hello, ${user.name}!`)
Arrow Functions
- Why it matters: Critical for Vue 3's Composition API.
- Used in setup() functions, computed properties, and watchers.
- Essential for maintaining correct this binding in methods.
const doubleCount = computed(() => count.value * 2) watch(() => user.name, (newValue, oldValue) => { console.log(`Name changed from ${oldValue} to ${newValue}`) })
Objects and Object Destructuring
- Why it matters: Fundamental for working with Vue's reactive objects.
- Required for component props and emits declarations.
- Essential for destructuring from setup() returns.
export default { setup(props, { emit }) { const { title, description } = props return { title, description } } }
Arrays and Array Methods
- Why it matters: Critical for rendering lists with v-for.
- Essential for reactive data manipulation.
- Used in computed properties for data transformation.
<template> <ul> <li v-for="item in filteredItems" :key="item.id">{{ item.name }}</li> </ul> </template> <script setup> const items = ref([/* ... */]) const filteredItems = computed(() => items.value.filter(item => item.isActive) ) </script>
Promises and Async/Await
- Why it matters: Crucial for data fetching in setup().
- Required for async component operations.
- Essential for lifecycle hooks and watchers.
import { onMounted } from 'vue' export default { async setup() { const data = ref(null) onMounted(async () => { data.value = await fetchData() }) return { data } } }
Modules and Exports
- Why it matters: Fundamental for component organization.
- Required for composables and plugins.
- Essential for maintaining clean architecture.
// useCounter.js import { ref } from 'vue' export function useCounter() { const count = ref(0) const increment = () => count.value++ return { count, increment } } // Component.vue import { useCounter } from './useCounter' export default { setup() { const { count, increment } = useCounter() return { count, increment } } }
Classes and Object-Oriented Concepts
- Why it matters: Helpful for understanding component inheritance.
- Used in custom directive implementations.
- Valuable for complex state management.
class BaseComponent { constructor(name) { this.name = name } sayHello() { console.log(`Hello from ${this.name}`) } } class SpecialComponent extends BaseComponent { constructor(name, special) { super(name) this.special = special } }
Optional Chaining
- Why it matters: Essential for safe property access in templates.
- Useful in computed properties.
- Helpful for handling async data states.
<template> <div>{{ user?.profile?.name }}</div> </template> <script setup> const user = ref(null) const userName = computed(() => user.value?.profile?.name ?? 'Guest') </script>
Event Handling
- Why it matters:Critical for component communication.
- Required for DOM event management.
- Essential for custom event implementations.
<template> <button>Click me</button> </template> <script setup> import { defineEmits } from 'vue' const emit = defineEmits(['custom-event']) function handleClick() { emit('custom-event', { data: 'Some data' }) } </script>
Error Handling
- Why it matters:Important for component error boundaries.
- Critical for API calls and async operations.
- Essential for maintaining app stability.
const count = ref(0) const user = reactive({ name: 'John', age: 30 })
These code snippets demonstrate practical applications of each concept within the context of Vue 3 development, providing concrete examples for developers to understand and apply these fundamental JavaScript skills.
Practical Applications of Core JavaScript Concepts
To illustrate how these essential JavaScript concepts are used in widely used beginner scenarios, let's explore three mini-projects: a weather app, a background color changer, and a todo app. These examples will demonstrate the practical application of the concepts we've discussed.
Weather App
const greeting = computed(() => `Hello, ${user.name}!`)
Core Concepts Implemented:
- Async/Await: For handling asynchronous API calls.
- Fetch API: To retrieve weather data from an external service.
- DOM Manipulation: To update the HTML content dynamically.
- Template Literals: For easy string interpolation and multi-line strings.
- Error Handling: Using try/catch to manage potential errors during the fetch operation.
Background Color Changer
const doubleCount = computed(() => count.value * 2) watch(() => user.name, (newValue, oldValue) => { console.log(`Name changed from ${oldValue} to ${newValue}`) })
Core Concepts Implemented:
- Arrow Functions: For concise function expressions.
- Math Object: To generate random RGB values for colors.
- Template Literals: For constructing the RGB string.
- Event Listeners: To handle user interactions (button clicks).
- DOM Manipulation: To change the background color and display the current color.
Todo App
export default { setup(props, { emit }) { const { title, description } = props return { title, description } } }
Core Concepts Implemented:
- Local Storage: For persisting todos across sessions.
- Array Methods: Using map for rendering and push/splice for modifying the todo list.
- Arrow Functions: For concise syntax in functions.
- Event Handling: To manage form submissions and button clicks.
- Template Literals: For generating HTML markup dynamically.
These mini-projects illustrate how core JavaScript concepts come together in practical applications. They showcase asynchronous programming, DOM manipulation, event handling, array methods, and more, providing a tangible context for understanding the above essential fundamental JavaScript skills before getting into Vue3.js development.
<template> <ul> <li v-for="item in filteredItems" :key="item.id">{{ item.name }}</li> </ul> </template> <script setup> const items = ref([/* ... */]) const filteredItems = computed(() => items.value.filter(item => item.isActive) ) </script>
The above is the detailed content of JavaScript Fundamentals for Vue Developers. For more information, please follow other related articles on the PHP Chinese website!
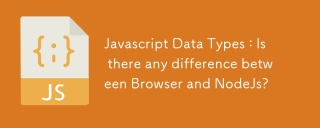
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
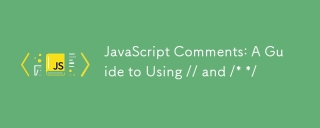
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
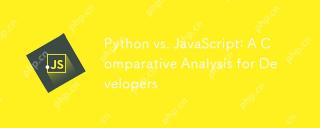
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
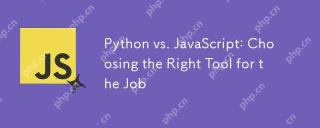
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
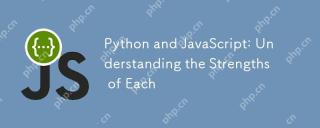
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
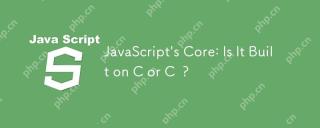
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
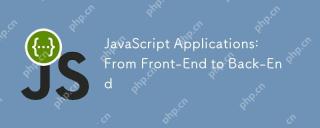
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
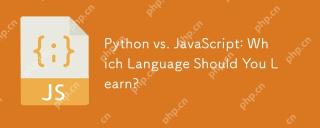
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
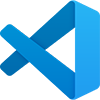
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
