


Passing Lists to SQL Server Stored Procedures from C#
In the given C# code, a stored procedure is being invoked with various parameter values, including a list of strings. However, the query within the stored procedure requires the list to be passed as part of the IN clause.
Solution: Using User Defined Table Types (SQL Server 2008 )
SQL Server 2008 introduced User Defined Table Types, a feature that allows passing collections of data to stored procedures in a structured manner. Here's how to implement this solution:
1. Create a User Defined Table Type
Create a table type that corresponds to the list of strings. For instance:
CREATE TYPE [dbo].[StringList] AS TABLE( [Item] [NVARCHAR](MAX) NULL );
2. Modify the Stored Procedure
Adapt the stored procedure to accept the table type as an input parameter:
CREATE PROCEDURE [dbo].[sp_UseStringList] @list StringList READONLY AS BEGIN -- Query using the table type SELECT l.Item FROM @list l; END
3. Populate the Table Type in C#
In C#, create a DataTable object and populate it with the list of strings. Then, define a parameter to pass the DataTable to the stored procedure.
using (var table = new DataTable()) { table.Columns.Add("Item", typeof(string)); for (int i = 0; i <p><strong>4. Execute the Stored Procedure</strong></p><p>Execute the stored procedure with the populated SqlParameter object.</p><p><strong>Example Usage:</strong></p><pre class="brush:php;toolbar:false">using (var con = new SqlConnection(connstring)) { con.Open(); using (SqlCommand cmd = new SqlCommand("exec sp_UseStringList @list", con)) { using (var dr = cmd.ExecuteReader()) { while (dr.Read()) Console.WriteLine(dr["Item"].ToString()); } } }
The above is the detailed content of How to Pass a C# String List to an SQL Server Stored Procedure's IN Clause?. For more information, please follow other related articles on the PHP Chinese website!
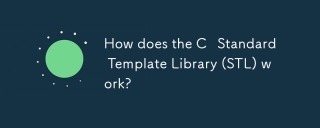
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
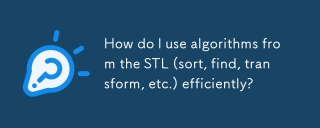
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
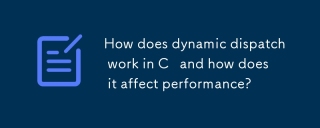
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
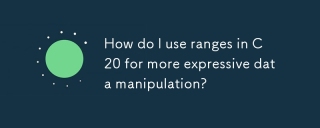
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
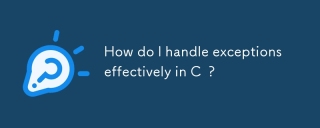
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
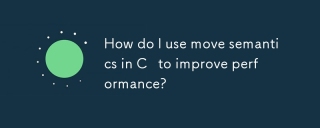
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
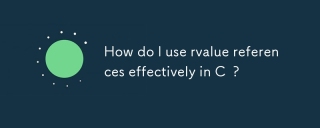
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
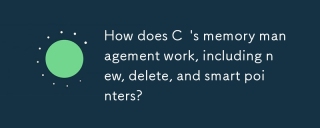
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
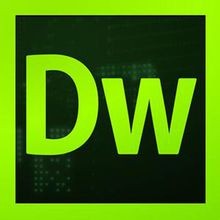
Dreamweaver CS6
Visual web development tools
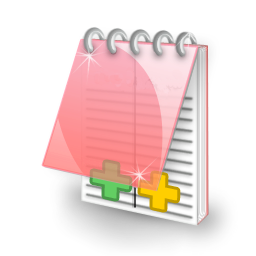
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
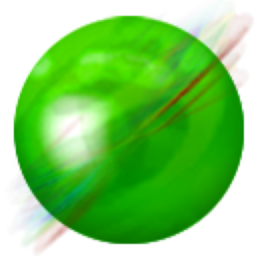
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
