Notify ObservableCollection When Item Changes
ObservableCollection is a handy collection type that implements INotifyCollectionChanged interface, which allows you to listen for changes in the collection. However, ObservableCollection does not notify about changes made to properties of the items within the collection.
Overcoming the Limitation
To address this limitation, you can implement a custom ObservableCollection called TrulyObservableCollection, which inherits from ObservableCollection and overrides the CollectionChanged event handler to subscribe and unsubscribe to the PropertyChanged event of items added and removed from the collection.
TrulyObservableCollection
public class TrulyObservableCollection<t> : ObservableCollection<t> where T : INotifyPropertyChanged { public TrulyObservableCollection() : base() { CollectionChanged += TrulyObservableCollection_CollectionChanged; } void TrulyObservableCollection_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e) { if (e.NewItems != null) { foreach (Object item in e.NewItems) { (item as INotifyPropertyChanged).PropertyChanged += new PropertyChangedEventHandler(item_PropertyChanged); } } if (e.OldItems != null) { foreach (Object item in e.OldItems) { (item as INotifyPropertyChanged).PropertyChanged -= new PropertyChangedEventHandler(item_PropertyChanged); } } } void item_PropertyChanged(object sender, PropertyChangedEventArgs e) { NotifyCollectionChangedEventArgs a = new NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction.Reset); OnCollectionChanged(a); } }</t></t>
Usage
To use TrulyObservableCollection, simply replace ObservableCollection with TrulyObservableCollection in your code. You need to attach handlers to the CollectionChanged event of the collection to handle changes.
MyItemsSource = new TrulyObservableCollection<mytype>(); MyItemsSource.CollectionChanged += MyItemsSource_CollectionChanged;</mytype>
Within the CollectionChanged event handler, you can subscribe to or unsubscribe from the PropertyChanged events of items added and removed from the collection.
void MyItemsSource_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e) { if (e.NewItems != null) foreach (MyType item in e.NewItems) item.PropertyChanged += new PropertyChangedEventHandler(MyType_PropertyChanged); if (e.OldItems != null) foreach (MyType item in e.OldItems) item.PropertyChanged -= new PropertyChangedEventHandler(MyType_PropertyChanged); }
Alternative Approach
While TrulyObservableCollection allows you to force collection change notifications, it may not be the most optimal approach. A more common pattern is to handle PropertyChanged events of individual items within the CollectionChanged handler of ObservableCollection. This ensures that only specific property changes within the collection cause UI updates, optimizing performance and providing more granular control.
The above is the detailed content of How to Make ObservableCollection Notify When Item Properties Change?. For more information, please follow other related articles on the PHP Chinese website!
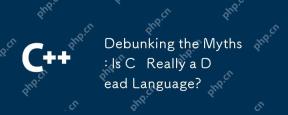
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
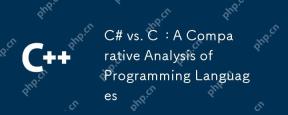
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
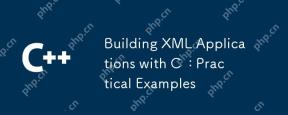
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
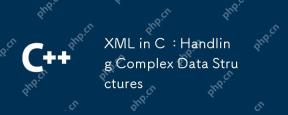
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
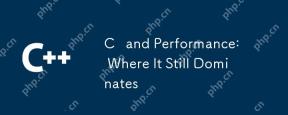
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
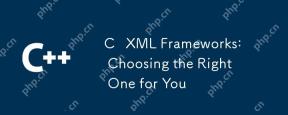
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
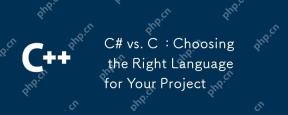
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
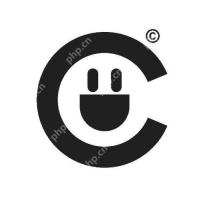
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
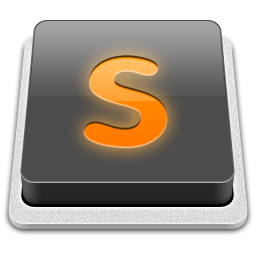
SublimeText3 Mac version
God-level code editing software (SublimeText3)
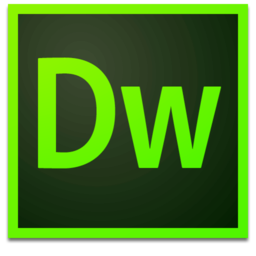
Dreamweaver Mac version
Visual web development tools
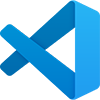
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
