Object Comparison in JavaScript
JavaScript presents challenges when comparing objects due to their inherent properties and prototype inheritance. Unlike primitive data types, objects are references to their respective instances. This means that == and === operators only yield true if both references point to the exact same object, failing to account for object equality with identical values.
To address this, various methods have been proposed:
1. JSON Stringification
This method utilizes JSON.stringify to convert both objects into JSON strings. These strings are then compared for equality:
JSON.stringify(obj1) === JSON.stringify(obj2)
However, this approach is sensitive to property order and may not accurately determine equality for objects with complex structures or circular references.
2. Deep Object Comparison
A more robust approach involves a recursive comparison algorithm that examines the objects' properties and their values:
function deepCompare(obj1, obj2) { // Check for object equality if (obj1 === obj2) return true; // Compare property order if (Object.keys(obj1).length !== Object.keys(obj2).length) return false; // Iterate over properties for (const key in obj1) { // Check property existence and type if (!obj2.hasOwnProperty(key) || typeof obj1[key] !== typeof obj2[key]) return false; // Recursive comparison for nested objects or functions if (typeof obj1[key] === 'object' || typeof obj1[key] === 'function') { if (!deepCompare(obj1[key], obj2[key])) return false; } else { // Compare primitive values if (obj1[key] !== obj2[key]) return false; } } return true; }
This algorithm thoroughly compares objects by traversing their properties recursively and verifying that they have the same structure and values.
It's worth noting that this is not a perfect solution, as some situations, such as objects with prototype inheritance or circular references, may still pose challenges. Nevertheless, this approach provides a comprehensive and reliable method for comparing objects in most scenarios.
The above is the detailed content of How Can I Reliably Compare Objects for Equality in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
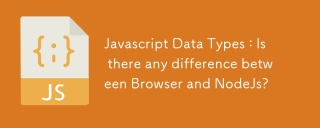
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
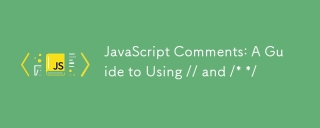
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
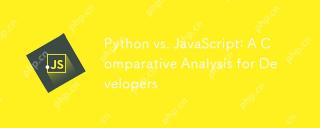
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
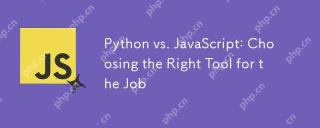
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
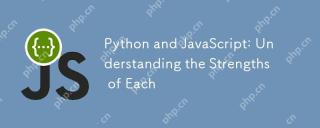
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
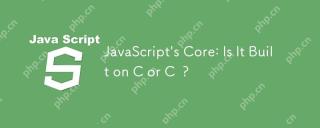
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
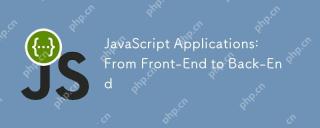
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
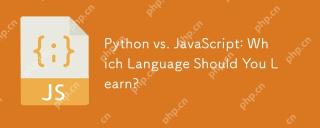
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
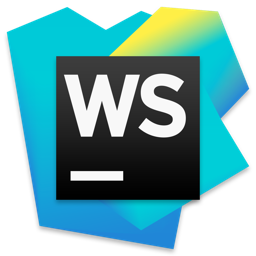
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
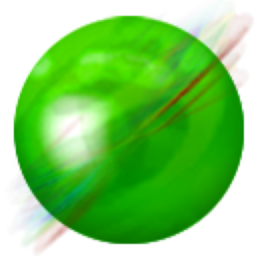
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
