


Pythonic Approaches to Implementing Getters and Setters
When defining and manipulating class properties in Python, it's essential to adhere to the language's best practices to enhance code readability and maintainability. Several options exist for implementing getters and setters, but the most Pythonic and idiomatic approach involves using the built-in property decorator.
The property decorator is a function decorator that allows you to define getters, setters, and deleters for a property. These functions are called when accessing, assigning, or deleting the property, respectively. The following example illustrates how to use the property decorator:
class C(object): def __init__(self): self._x = None @property def x(self): """I'm the 'x' property.""" print("getter of x called") return self._x @x.setter def x(self, value): print("setter of x called") self._x = value @x.deleter def x(self): print("deleter of x called") del self._x c = C() c.x = 'foo' # setter called foo = c.x # getter called del c.x # deleter called
In this example, the x property has a getter, setter, and deleter defined using the @property, @x.setter, and @x.deleter decorators, respectively. When you access the x property through c.x, the getter is called. Similarly, when you assign to the x property through c.x = 'foo', the setter is called. Finally, when you delete the x property through del c.x, the deleter is called.
This approach provides a clean and concise way to implement getters and setters in Python, adhering to the language's philosophy of encapsulation and data hiding. By using the property decorator, you can define custom logic for accessing, modifying, or deleting properties, ensuring that the underlying data remains protected.
The above is the detailed content of How Can I Pythonically Implement Getters and Setters for Class Properties?. For more information, please follow other related articles on the PHP Chinese website!
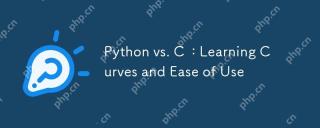
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
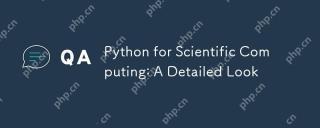
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
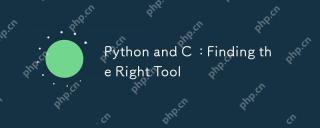
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
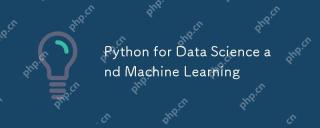
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
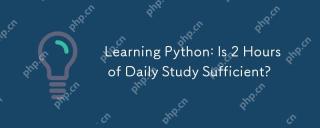
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
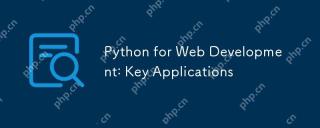
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
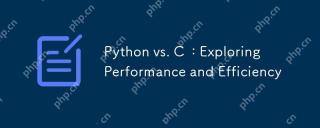
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
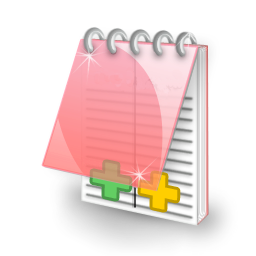
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
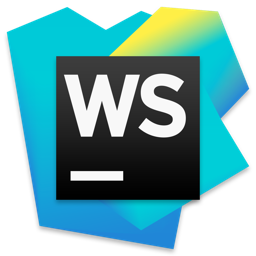
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.