


Returning Multiple Table Fields as a Record in PostgreSQL with PL/pgSQL
When working with PL/pgSQL, returning multiple fields from several tables as a record can be achieved by employing the RECORD data type. This allows for the creation of a composite data type that can hold fields with different data types.
Example Code
Consider the following modified example, which addresses the additional complexity of retrieving fields from separate rows in a table:
CREATE TABLE user (id int, school_id int, name varchar(32)); CREATE TYPE my_type AS ( user1_id int, user1_name varchar(32), user2_id int, user2_name varchar(32) ); CREATE OR REPLACE FUNCTION get_two_users_from_school(schoolid int) RETURNS my_type AS $$ DECLARE result my_type; temp_result user; BEGIN SELECT id, name INTO temp_result FROM user WHERE school_id = schoolid ORDER BY id ASC LIMIT 2; result.user1_id := temp_result[1].id; result.user1_name := temp_result[1].name; IF temp_result[2].id IS NOT NULL THEN result.user2_id := temp_result[2].id; result.user2_name := temp_result[2].name; END IF; RETURN result; END $$ language plpgsql;
In this example, the get_two_users_from_school function returns a my_type record with four fields: user1_id, user1_name, user2_id, and user2_name. It retrieves and organizes the data from the user table, flattening the rows into a single, cohesive record.
Dynamic Record Structure
Note that the returned record is not strictly limited to a specific number of fields. You can return an arbitrary number of fields based on the data retrieved from the database.
Usage
To use the function, you can execute the following query:
SELECT * FROM get_two_users_from_school(123);
This will return a record with user details from the specified school ID.
The above is the detailed content of How to Return Multiple Table Fields as a Single Record in PostgreSQL using PL/pgSQL?. For more information, please follow other related articles on the PHP Chinese website!
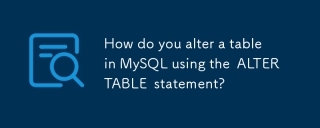
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
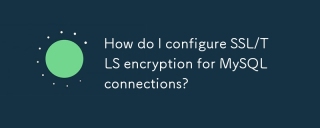
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
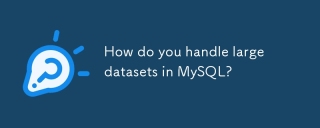
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
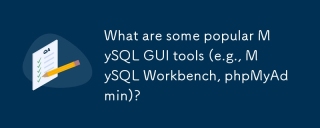
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
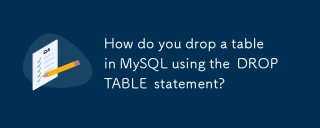
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
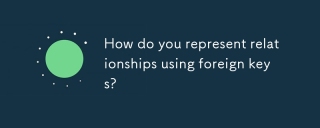
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
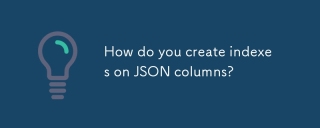
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
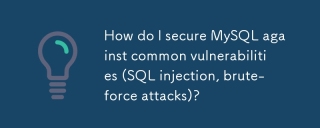
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
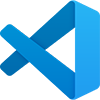
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
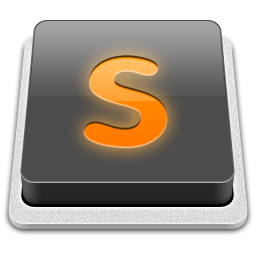
SublimeText3 Mac version
God-level code editing software (SublimeText3)
