


How Can Pixel Outlines Enable Efficient Collision Detection in Complex Game Environments?
Collision Detection with Complex Shapes using Pixel Outlines
In order to create a game that allows players to interact with a complex, non-rectangular environment, it is necessary to implement a collision detection system that can handle intricate shapes.
One effective approach is to utilize pixel outlines as the collision boundaries. By identifying the outer edges of the desired collision area, such as the red pixels in a background image, an accurate representation of the shape can be established.
Here's a sample Java code snippet that demonstrates the idea:
import java.awt.*; import java.awt.geom.*; import java.awt.image.BufferedImage; class ShapeCollision { private BufferedImage img; private Area obstacle; int x; int y; int xDelta = 3; int yDelta = 2; public boolean doAreasCollide(Area area1, Area area2) { // Implementation omitted for brevity } ShapeCollision(BufferedImage srcImage) { img = srcImage; // Get the outline of the red pixels obstacle = getOutline(Color.RED, img); // Initialize the player's position x = img.getWidth() / 2; y = img.getHeight() / 2; } private Area getOutline(Color target, BufferedImage bi) { // Implementation omitted for brevity } public void animate() { Graphics2D g = img.createGraphics(); ... // Collision detection logic omitted for brevity ... g.dispose(); } public static void main(String[] args) { // Load the source image BufferedImage srcImage = ... // Create the ShapeCollision instance ShapeCollision shapeCollision = new ShapeCollision(srcImage); ... } }
In this code, the obstacle variable represents the collision boundary, which is derived from the outline of the red pixels in the srcImage during the initialization of ShapeCollision.
By leveraging pixel outlines, this approach provides a flexible and computationally efficient way to detect collisions with complex shapes, enabling the creation of dynamic and immersive game environments.
The above is the detailed content of How Can Pixel Outlines Enable Efficient Collision Detection in Complex Game Environments?. For more information, please follow other related articles on the PHP Chinese website!
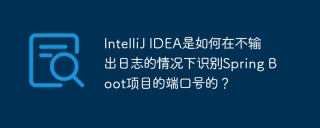
Start Spring using IntelliJIDEAUltimate version...
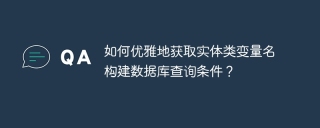
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
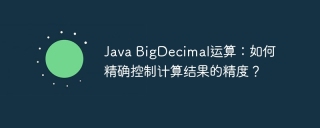
Java...
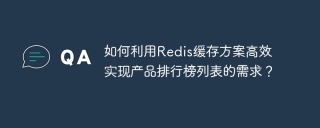
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
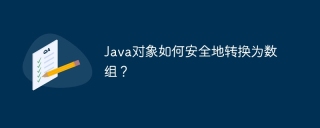
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
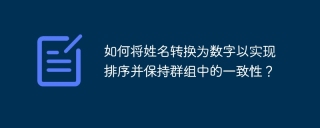
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
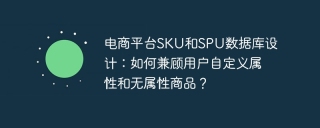
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
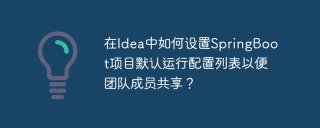
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
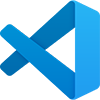
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
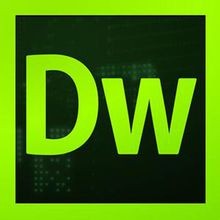
Dreamweaver CS6
Visual web development tools