Printing Struct Values with Pointers in Go
In Go, it's common to encounter situations where you need to print the value of a struct that contains pointers to other structs. However, the default behavior of the %v format specifier in fmt.Printf() displays the pointer address instead of the actual value.
Consider the following example:
package main import "fmt" type A struct { a int32 B *B } type B struct { b int32 } func main() { a := &A{ a: 1, B: &B{ b: 2, }, } fmt.Printf("v ==== %+v \n", a) }
The output of the above code is:
v ==== &{a:1 B:0xc42000e204}
As you can see, the B field is printed as the memory address of the B struct, not its actual value.
Custom Printing with Stringer Interface
One way to print the contents of nested structs is to implement the Stringer interface for both the A and B types. The Stringer interface requires a single method, String(), which returns a string representation of the value.
Here's an updated example with the Stringer interface:
package main import "fmt" type A struct { a int32 B *B } type B struct{ b int32 } func (aa *A) String() string { return fmt.Sprintf("A{a:%d, B:%v}",aa.a,aa.B) } func (bb *B) String() string { return fmt.Sprintf("B{b:%d}",bb.b) } func main() { a := &A{a: 1, B: &B{b: 2}} // using the Stringer interface fmt.Printf("v ==== %v \n", a) // or just print it yourself however you want. fmt.Printf("v ==== A{a:%d, B:B{b:%d}}\n", a.a, a.B.b) // or just reference the values in the struct that are structs themselves // but this can get really deep fmt.Printf("v ==== A{a:%d, B:%v}", a.a, a.B) }
Now, the output is:
v ==== A{a:1, B:B{b:2}}
Manual Printing
If you don't wish to implement the Stringer interface, you can manually print the desired representation of the struct using the print statements. For example, you can access the nested struct's fields and print them separately:
fmt.Printf("v ==== A{a:%d, B:B{b:%d}}\n", a.a, a.B.b)
This approach gives you complete control over the format of the output.
The above is the detailed content of How to Print the Values of Nested Structs with Pointers in Go?. For more information, please follow other related articles on the PHP Chinese website!
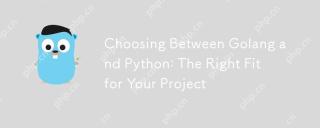
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
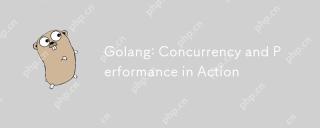
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
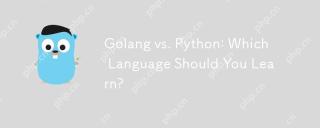
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
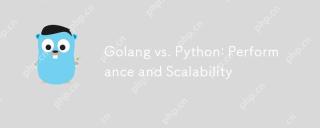
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
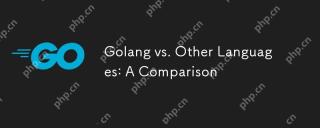
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
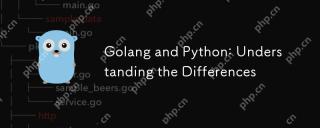
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
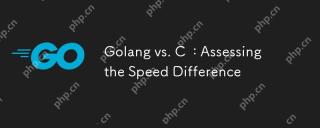
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
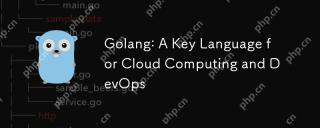
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
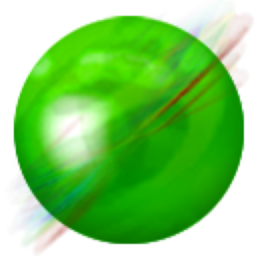
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor