


Analyzing Code Performance Issues
In this code, slow performance arises from the expensive heuristic computation within the astar function. To enhance performance, consider the following:
Realtime Performance Monitoring
As demonstrated in the analysis, profiling tools like stack sampling can quickly identify performance bottlenecks. By examining the stack traces, you can pinpoint statements that consume excessive time.
The Heuristic Function
The heuristic function, heuristic, unnecessarily loops over the entire formation array, resulting in significant overhead. A more efficient approach is to maintain a running sum of fCamel and bCamel while traversing the array.
def heuristic(formation): fCamels, bCamels = 0, 0 for i in formation: if i == fCamel: fCamels += 1 elif i == bCamel: bCamels += fCamels * bCamels # Update to fCamel * bCamel differences else: pass return bCamels
Optimizing the A* Algorithm
Within the astar function, the openlist is a priority queue that sorts nodes based on their f values. The openlist.put call incurs unnecessary overhead because the f values are already computed and stored in the node objects.
A more efficient approach is to override the __lt__ operator for the node class to directly compare the f values. This eliminates the need for the f parameter in openlist.put.
def __lt__(self, other): return self.f <p>Additionally, ensure that the open list is maintained in ascending order of the f values, as required by the A* algorithm. The default implementation in the Queue module does not guarantee this behavior.</p>
The above is the detailed content of How Can We Optimize A* Algorithm Performance by Improving Heuristic Function and Priority Queue Management?. For more information, please follow other related articles on the PHP Chinese website!
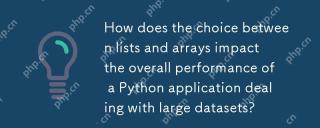
ForhandlinglargedatasetsinPython,useNumPyarraysforbetterperformance.1)NumPyarraysarememory-efficientandfasterfornumericaloperations.2)Avoidunnecessarytypeconversions.3)Leveragevectorizationforreducedtimecomplexity.4)Managememoryusagewithefficientdata
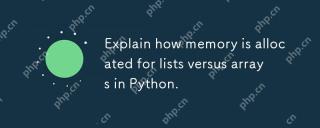
InPython,listsusedynamicmemoryallocationwithover-allocation,whileNumPyarraysallocatefixedmemory.1)Listsallocatemorememorythanneededinitially,resizingwhennecessary.2)NumPyarraysallocateexactmemoryforelements,offeringpredictableusagebutlessflexibility.
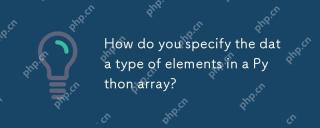
InPython, YouCansSpectHedatatYPeyFeLeMeReModelerErnSpAnT.1) UsenPyNeRnRump.1) UsenPyNeRp.DLOATP.PLOATM64, Formor PrecisconTrolatatypes.
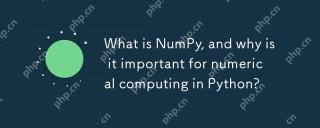
NumPyisessentialfornumericalcomputinginPythonduetoitsspeed,memoryefficiency,andcomprehensivemathematicalfunctions.1)It'sfastbecauseitperformsoperationsinC.2)NumPyarraysaremorememory-efficientthanPythonlists.3)Itoffersawiderangeofmathematicaloperation
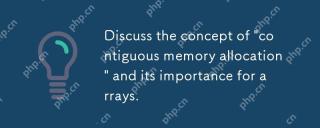
Contiguousmemoryallocationiscrucialforarraysbecauseitallowsforefficientandfastelementaccess.1)Itenablesconstanttimeaccess,O(1),duetodirectaddresscalculation.2)Itimprovescacheefficiencybyallowingmultipleelementfetchespercacheline.3)Itsimplifiesmemorym
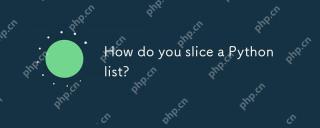
SlicingaPythonlistisdoneusingthesyntaxlist[start:stop:step].Here'showitworks:1)Startistheindexofthefirstelementtoinclude.2)Stopistheindexofthefirstelementtoexclude.3)Stepistheincrementbetweenelements.It'susefulforextractingportionsoflistsandcanuseneg

NumPyallowsforvariousoperationsonarrays:1)Basicarithmeticlikeaddition,subtraction,multiplication,anddivision;2)Advancedoperationssuchasmatrixmultiplication;3)Element-wiseoperationswithoutexplicitloops;4)Arrayindexingandslicingfordatamanipulation;5)Ag
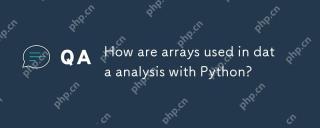
ArraysinPython,particularlythroughNumPyandPandas,areessentialfordataanalysis,offeringspeedandefficiency.1)NumPyarraysenableefficienthandlingoflargedatasetsandcomplexoperationslikemovingaverages.2)PandasextendsNumPy'scapabilitieswithDataFramesforstruc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
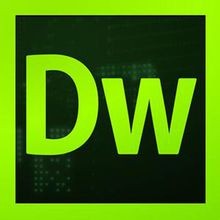
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
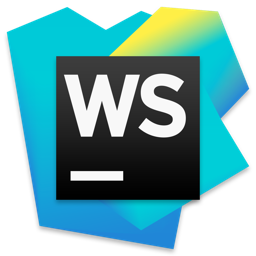
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
