Checking the Checkbox's Checked Property with jQuery
Problem:
In jQuery, you are trying to check the checked property of a checkbox to perform an action based on its status. However, the code isn't working correctly, and it's returning false as the default value.
Solution:
To successfully query the checked property of a checkbox in jQuery, follow these steps:
-
Use the jQuery Attribute Selector:
Instead of using the attr() method, use the attribute selector syntax to retrieve the checked property directly. The attribute selector can be applied to the checkbox's ID or name attribute.
-
Check the Checked Value:
Once you have the checked property, compare its value to true to determine if the checkbox is checked. The following code example shows how to do this:
if (jQuery('#isAgeSelected').prop('checked')) { // Checkbox is checked // Show the textbox } else { // Checkbox is unchecked // Hide the textbox }
-
Toggle the Element's Visibility:
To hide or show the element based on the checkbox's checked status, use jQuery's toggle() method. This method takes a boolean value and toggles the visibility of the element accordingly.
-
Event-Based Approach:
Alternatively, you can use an event-driven approach to update the element's visibility when the checkbox state changes. Attach a change event handler to the checkbox and use the toggle() method to update the element's visibility within the handler.
Example Code:
// Check the checked property on page load if ($('#isAgeSelected').prop('checked')) { $("#txtAge").show(); } else { $("#txtAge").hide(); } // Event-driven approach $('#isAgeSelected').change(function() { $("#txtAge").toggle(this.checked); });
The above is the detailed content of How Do I Correctly Check a Checkbox's Checked Property in jQuery?. For more information, please follow other related articles on the PHP Chinese website!
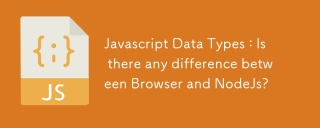
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
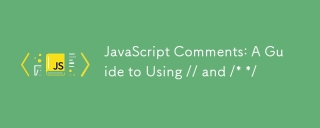
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
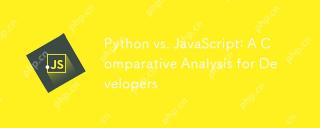
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
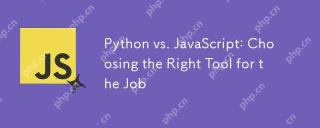
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
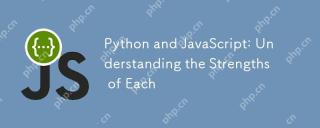
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
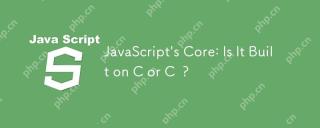
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
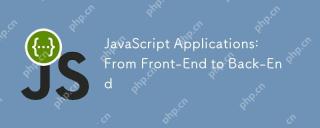
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
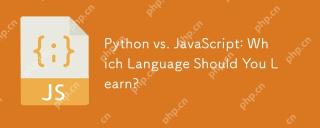
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
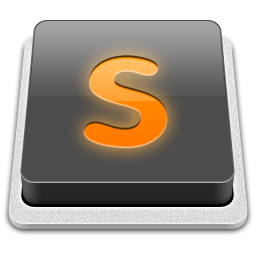
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
