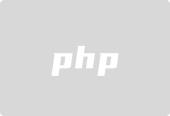
Copying to the Clipboard in JavaScript
General Overview
To copy text to the clipboard in JavaScript, there are three key APIs:
-
Async Clipboard API (navigator.clipboard.writeText):
- Primary API for Chrome 66 and above (March 2018).
- Asynchronous access using JavaScript Promises, ensuring a smooth user experience.
- Text can be copied directly from a variable.
- Requires HTTPS for accessing the clipboard.
-
document.execCommand('copy'):
- Deprecated but widely supported.
- Synchronous access, halting JavaScript execution until copying is complete.
- Text is read from the DOM and placed on the clipboard.
- May display security prompts during testing in the console.
-
Overriding the Copy Event:
- Allows modification of clipboard data from any copy event.
- Supports multiple data formats beyond plain text.
Development Notes
- Clipboard-related commands may not work in console tests. Page interaction or an active tab is often required.
- Due to browser deprecation of cross-origin permissions in IFRAMEs, embedded demos may not work in browsers like Chrome and Microsoft Edge.
Async Plus Fallback
Combining the Async Clipboard API with a fallback to document.execCommand('copy') ensures wide browser support:
function copyTextToClipboard(text) {
if (!navigator.clipboard) {
fallbackCopyTextToClipboard(text);
return;
}
navigator.clipboard.writeText(text).then(function() {
console.log('Async: Copying to clipboard was successful!');
}, function(err) {
console.error('Async: Could not copy text: ', err);
});
}
The above is the detailed content of How Can I Efficiently Copy Text to the Clipboard in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn