


Member Initializer Lists vs. Constructor Assignments: A Deeper Dive
In C , it is often debated whether there is a significant difference between using a member initializer list and assigning values in a constructor. While both approaches achieve the same end result, there are subtle distinctions that may be important in specific cases.
Member Initializer List: A Glance
A member initializer list is a comma-separated list of member names and their initial values, enclosed in curly braces and placed at the beginning of a constructor. For instance:
class MyClass { private: int _capacity; int* _data; int _len; public: MyClass(): _capacity(15), _data(NULL), _len(0) {} };
Here, the constructor initializes all three member variables in the member initializer list.
Assignments in Constructor: A Different Approach
Alternatively, one can assign values to members within the constructor body, as seen here:
class MyClass { private: int _capacity; int* _data; int _len; public: MyClass() { _capacity = 15; _data = NULL; _len = 0; } };
In this case, the constructor initializes members using individual statements.
The Internal Perspective: Code Generation
When compiled, both approaches ultimately result in the same machine code. The compiler generates code to assign values to member variables in both cases, regardless of whether an initializer list is used.
Key Differences: When Initialization Matters
While functionally equivalent, member initializer lists and constructor assignments differ primarily in circumstances where initializing members presents specific requirements:
- Constant Members: When initializing constant members (declared with const), a member initializer list is mandatory, as it ensures immediate initialization.
- References: References must be initialized in the initializer list.
- Base Class Parameters: When a base class constructor requires parameters, they should be passed through the initializer list.
Conclusion
While member initializer lists and constructor assignments may seem interchangeable, their distinction lies in specific scenarios where exact initialization order or immutability (constant members) is crucial. In such cases, member initializer lists provide a clear and reliable mechanism for initializing members.
The above is the detailed content of Member Initializer Lists vs. Constructor Assignments: When Does It Matter?. For more information, please follow other related articles on the PHP Chinese website!
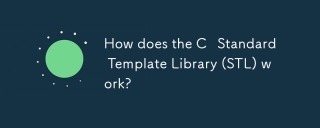
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
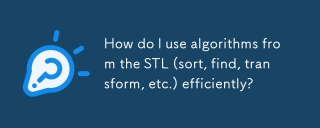
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
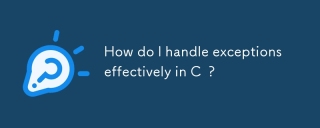
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
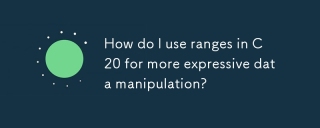
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
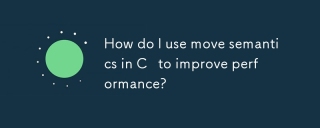
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
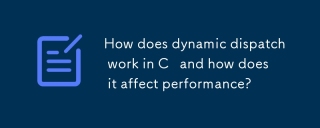
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
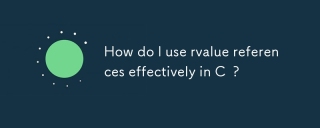
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
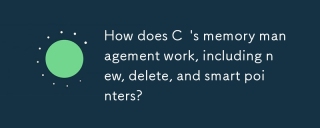
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
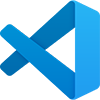
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft