


Pagination through MySQL LIMIT and OFFSET
Challenges of Hardcoded Pagination
When creating paginated results, it can be cumbersome to manually hardcode each page's offset. This approach becomes impractical when dealing with a large number of items.
Dynamic Pagination with URL Parameters
A more efficient solution is to dynamically generate pagination via URL parameters. By passing the requested page number through the URL (e.g., http://yoursite.com/itempage.php?page=2), you can easily calculate the offset for the associated query.
$page = isset($_GET['page']) ? filter_input(INPUT_GET, 'page', FILTER_VALIDATE_INT) : 1;
Calculating Total Pages from Row Count
To enable user navigation across all result pages, it's essential to determine the total number of pages based on the table's row count.
$sql = "SELECT COUNT(*) FROM menuitem"; $result = mysqli_query($con, $sql); $row_count = mysqli_num_rows($result); $page_count = ceil($row_count / $items_per_page);
Creating the Pagination Query
Now, with the requested page number and total page count, you can formulate the SQL query with the appropriate LIMIT and OFFSET.
$offset = ($page - 1) * $items_per_page; $sql = "SELECT * FROM menuitem LIMIT $offset, $items_per_page";
Generating Page Links
Finally, to provide navigation links for users, you can dynamically create links for each page with the appropriate URL parameters.
for ($i = 1; $i <p><strong>Benefits of Dynamic Pagination</strong></p><p>This approach eliminates the need for hardcoded pages, simplifies database queries, and provides a flexible user experience for navigating paginated data. It scales well to handle a large number of records and ensures consistency in pagination behavior across your application.</p>
The above is the detailed content of How Can I Efficiently Implement Dynamic Pagination in MySQL Using LIMIT and OFFSET?. For more information, please follow other related articles on the PHP Chinese website!
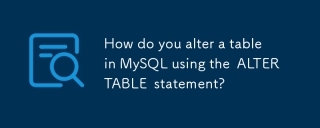
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
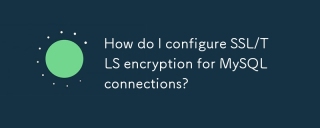
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
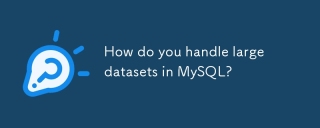
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
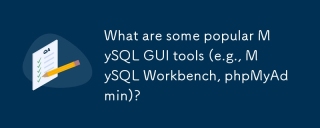
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
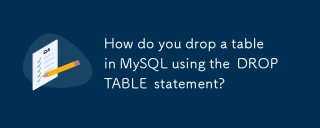
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
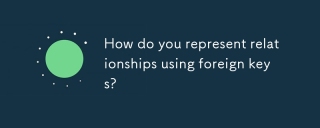
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
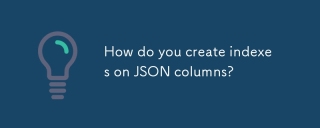
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
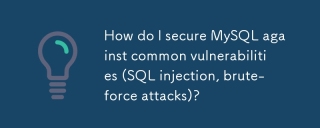
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
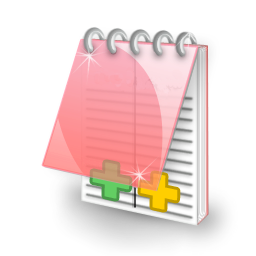
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
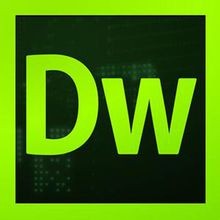
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
