


Why Does Java's Integer Boxing Produce Different Results for `Integer == Integer` Comparisons?
Weird Java Integer Boxing
Java's integer boxing mechanism can leave you slightly confused, especially when comparing reference equality.
Problem
Consider the following code snippet:
public class Scratch { public static void main(String[] args) { Integer a = 1000, b = 1000; System.out.println(a == b); // false Integer c = 100, d = 100; System.out.println(c == d); // true } }
When run, it will generate:
false true
First line The result is expected because a and b are different objects. But what is the result of the second line?
Answer
The true result of the second line is guaranteed by the language specification. According to Section 5.1.7:
If the value p to be boxed is true, false, byte or char in the range u0000 to u007f, or int or short between -128 and 127, then The results of any two boxing transformations on p, r1 and r2, are always equal.
While the second line of output is guaranteed, the first line is not (see the last paragraph quoted below):
Ideally, given a primitive value p Boxing always generates the same reference. In practice, this may not be possible using existing implementation techniques. The above rule is a pragmatic compromise. The last clause above requires that certain common values be always boxed into indistinguishable objects. Implementations MAY cache it, either lazily or immediately.
For other values, this representation does not allow the programmer to make any assumptions about the identity of the boxed value. This allows (but does not force) sharing of some or all of these references.
This ensures that in most common cases the behavior will be the desired behavior without unnecessary loss of performance, especially on small devices. Less memory-constrained implementations can cache all characters and shorts, as well as integers and longs in the range -32K to 32K.
The above is the detailed content of Why Does Java's Integer Boxing Produce Different Results for `Integer == Integer` Comparisons?. For more information, please follow other related articles on the PHP Chinese website!
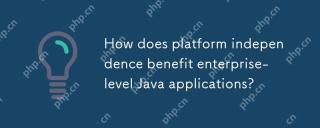
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
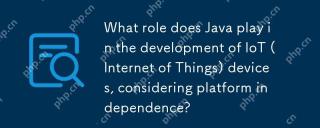
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
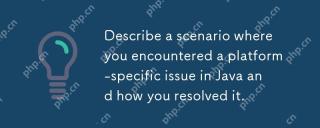
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
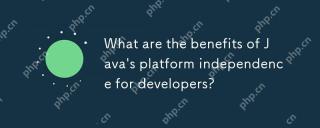
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
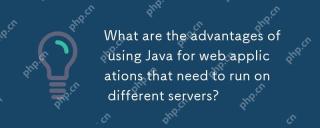
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
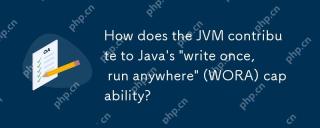
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
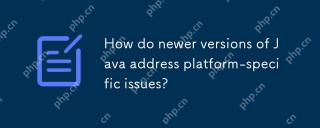
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
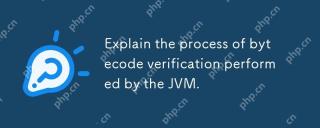
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
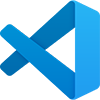
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
