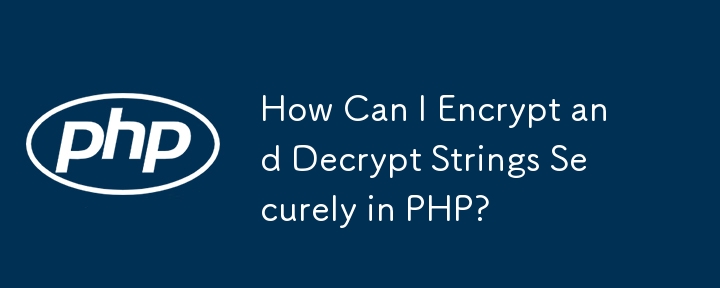
How to Encrypt and Decrypt a PHP String with Key
Encrypting and decrypting strings in PHP involves utilizing cryptographic techniques to protect sensitive information.
Encryption
To encrypt a string using a secret key:
-
Use AES in CTR mode: Encrypt the string using the Advanced Encryption Standard (AES) in Counter (CTR) mode.
-
Authenticate the ciphertext: Calculate a Hash-based Message Authentication Code (HMAC) over the IV and ciphertext using HMAC-SHA-256 or Poly1305 (for stream ciphers).
Decryption
To decrypt an encrypted string using the same key:
-
Check the MAC: Recalculate the MAC and compare it to the saved one.
-
Decrypt the message: Decrypt the ciphertext using AES in CTR mode.
Other Considerations
- Avoid compressing data before encryption.
- Use mb_strlen() and mb_substr() with the '8bit' character set to prevent issues.
- Generate IVs using a CSPRNG and avoid MCRYPT_RAND.
- Always encrypt then MAC to ensure both confidentiality and authenticity.
Libsodium for PHP
If using PHP 7.2 or higher, consider using the libsodium library:
use ParagonIE\Halite\Symmetric\Crypto as SymmetricCrypto;
$key = random_bytes(SODIUM_CRYPTO_SECRETBOX_KEYBYTES);
$ciphertext = SymmetricCrypto::encrypt($message, $key);
$plaintext = SymmetricCrypto::decrypt($ciphertext, $key);
defuse/php-encryption
As an alternative to libsodium:
use Defuse\Crypto\Crypto;
$key = Key::createNewRandomKey();
$ciphertext = Crypto::encrypt($message, $key);
$plaintext = Crypto::decrypt($ciphertext, $key);
Password Considerations
-
For passwords: Hash them using Argon2, scrypt, bcrypt, or PBKDF2-SHA256 with high iterations.
-
For authentication: Use HMAC-based algorithms like HKDF2 or hash_hmac() instead of encryption.
-
For URL parameters: Use an appropriate framework or library designed for URL parameter validation and tampering protection.
The above is the detailed content of How Can I Encrypt and Decrypt Strings Securely in PHP?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn