Redux Basics: A Detailed Explanation with Code Examples
Redux is a state management library used widely with JavaScript applications, especially with React. It provides a centralized store for the state of your app, making it easier to manage and debug, especially in large and complex applications. Redux follows a unidirectional data flow and ensures that state changes happen in a predictable manner, making it easier to understand how your app works.
Let's break down the Redux basics step by step, explaining each concept with code examples.
1. What is Redux?
Redux is a predictable state container for JavaScript apps. It helps you manage the state of your app in a centralized way, making it easier to debug and scale.
Core Principles of Redux:
- Single Source of Truth: The whole state of the application is stored in a single object (the store), making it easy to track and manipulate.
- State is Read-Only: The only way to change the state is by dispatching an action.
- Changes are Made with Pure Functions: State is modified by reducers, which are pure functions that specify how the state changes in response to an action.
2. Core Concepts of Redux
Redux relies on the following key components:
1. Action
An action is a plain JavaScript object that describes an event or an action that has occurred in the application. Each action must have a type property, which describes the action being performed.
Action Example:
// actions.js export const increment = () => ({ type: 'INCREMENT' }); export const decrement = () => ({ type: 'DECREMENT' });
- Here, increment and decrement are action creators. They return action objects with a type field. The type property tells Redux how to handle the action in the reducer.
2. Reducer
A reducer is a pure function that takes the current state and an action, then returns a new state. Reducers are the functions that specify how the state should change in response to an action. They should be pure functions, meaning they don’t modify the original state but return a new state object.
Reducer Example:
// actions.js export const increment = () => ({ type: 'INCREMENT' }); export const decrement = () => ({ type: 'DECREMENT' });
- In the example above:
- The initial state is defined with a count of 0.
- The reducer listens for actions INCREMENT and DECREMENT and updates the count state accordingly.
- The return { ...state, count: state.count 1 } line creates a new object to maintain immutability and update the state.
3. Store
The store holds the entire state of your application. The store is created using the createStore method from Redux, and it is where the application’s state lives. The store also provides methods to dispatch actions and subscribe to changes in the state.
Store Example:
// reducer.js const initialState = { count: 0 }; const counterReducer = (state = initialState, action) => { switch (action.type) { case 'INCREMENT': return { ...state, count: state.count + 1 }; case 'DECREMENT': return { ...state, count: state.count - 1 }; default: return state; } }; export default counterReducer;
- The store is created by passing the counterReducer to createStore.
- Now, the state of the application is managed by Redux, and any changes to the state will go through the reducer.
3. Connecting Redux with React
React components need to interact with the Redux store to get the state and dispatch actions. React-Redux, a separate library, is used to connect React with Redux. It provides hooks like useSelector to access the store’s state and useDispatch to dispatch actions.
Steps to Connect Redux with React:
- Wrap your app with the Provider component from react-redux to pass the store to all your components.
- Use useSelector to access the state.
- Use useDispatch to dispatch actions that modify the state.
4. Setting Up Redux in a React App
Let’s walk through the complete setup to connect Redux with a simple React app.
Step 1: Install Redux and React-Redux
First, you need to install Redux and React-Redux:
// store.js import { createStore } from 'redux'; import counterReducer from './reducer'; const store = createStore(counterReducer); export default store;
Step 2: Create Actions
In Redux, actions are plain JavaScript objects that describe the change you want to make to the state.
npm install redux react-redux
- The increment and decrement functions are action creators that return action objects.
- The action object has a type field that describes the action.
Step 3: Create a Reducer
A reducer is a function that takes the current state and an action, and returns a new state.
// actions.js export const increment = () => ({ type: 'INCREMENT' }); export const decrement = () => ({ type: 'DECREMENT' });
- The reducer listens for the INCREMENT and DECREMENT actions and updates the state accordingly.
Step 4: Create the Store
The store is where the state lives. It is created using the createStore method from Redux.
// actions.js export const increment = () => ({ type: 'INCREMENT' }); export const decrement = () => ({ type: 'DECREMENT' });
- We pass the counterReducer to createStore to create a store that will manage the state for our app.
Step 5: Connecting Redux with React
Now, let's connect Redux to our React app using the Provider, useDispatch, and useSelector hooks.
// reducer.js const initialState = { count: 0 }; const counterReducer = (state = initialState, action) => { switch (action.type) { case 'INCREMENT': return { ...state, count: state.count + 1 }; case 'DECREMENT': return { ...state, count: state.count - 1 }; default: return state; } }; export default counterReducer;
- Provider: Wraps the entire application, passing the Redux store down to all components.
- useSelector: Retrieves the current state (count) from the Redux store.
- useDispatch: Allows you to dispatch actions (increment and decrement).
5. Redux Toolkit (Optional)
To make Redux easier to use, Redux Toolkit simplifies setup by reducing boilerplate code. It offers utilities like createSlice and configureStore to handle common tasks such as creating reducers and configuring the store.
Example Using Redux Toolkit:
// store.js import { createStore } from 'redux'; import counterReducer from './reducer'; const store = createStore(counterReducer); export default store;
- The createSlice function automatically generates action creators and reducers for you.
- configureStore simplifies store setup.
6. Best Practices for Using Redux
- Keep Redux for Global State: Use Redux for managing application-wide state (e.g., user authentication, settings).
- Use Local State for Simple Components: For smaller state needs (like form inputs), use React's useState instead of Redux.
- Avoid Direct Mutation: Always return a new object when updating state to ensure immutability.
7. Conclusion
Redux is a powerful tool for managing global state in React applications. By understanding actions, reducers, and the store, you can manage complex state in a predictable way. Using Redux Toolkit can simplify this process further. When used correctly, Redux can make large applications easier to manage, debug, and scale.
With this knowledge, you're now equipped to incorporate Redux into your React applications, ensuring more efficient state management across your app.
The above is the detailed content of Mastering Redux Basics: A Complete Guide to State Management in React. For more information, please follow other related articles on the PHP Chinese website!
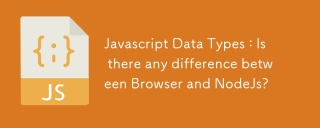
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
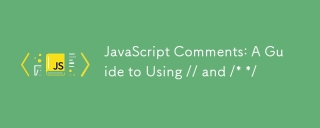
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
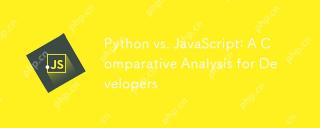
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
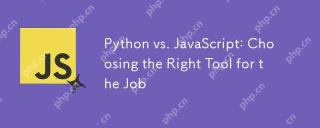
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
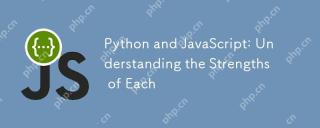
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
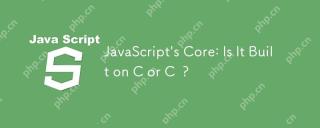
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
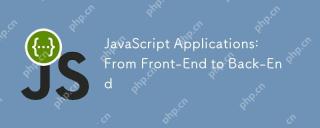
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
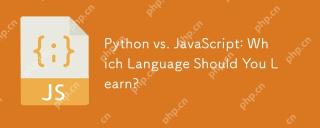
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
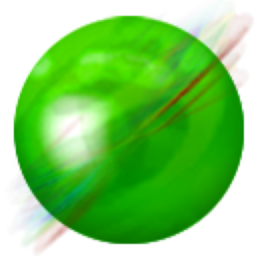
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
