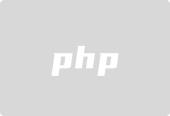
Understanding the Significance of (Star/Asterisk) and * (Double Star/Asterisk) in Parameters
In Python function definitions, the and * operators provide powerful idioms for handling arbitrary numbers of arguments.
Positional Arguments: *args
- The *args parameter allows a function to accept a variable number of positional arguments.
- These arguments are collected into a tuple that can be accessed within the function.
Example:
def foo(x, y, *args):
pass
foo(1, 2, 3)
# args = (3,)
Keyword Arguments: kwargs**
- The **kwargs parameter allows a function to accept any number of keyword arguments.
- These arguments are collected into a dictionary where the keys are the argument names and the values are the argument values.
Example:
def bar(x, y, **kwargs):
pass
bar(x=1, y=2, name='John', age=27)
# kwargs = {'name': 'John', 'age': 27}
Mixing Fixed and Variable Arguments
- Both args and *kwargs can be combined with normal fixed arguments to provide flexibility in function definitions.
Example:
def foo(kind, *args, bar=None, **kwargs):
print(kind, args, bar, kwargs)
foo(123, 'a', 'b', apple='red')
# 123 ('a', 'b') None {'apple': 'red'}
Additional Uses
- can be used to unpack argument lists when calling a function.
- In Python 3, and * can be used on the left side of an assignment to unpack iterables into variables.
- Python 3.6 introduces a new syntax that restricts positional arguments after *.
Note:
- Python uses dictionaries to represent keyword arguments, which maintain insertion order in Python 3.6 .
-
kwargs dictionaries maintain insertion order in Python 3.7 and above, even for non-CPython implementations.
The above is the detailed content of How Do `*args` and `kwargs` Handle Variable Arguments in Python Functions?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn