


How Does Go Achieve Lambda Expression Functionality Without Explicit Support?
Lambda Expressions in Go: A Detailed Explanation
Lambda expressions, a convenient feature in many modern languages, allow developers to define anonymous functions on the fly. While Go doesn't explicitly support lambda expressions, it provides a similar construct that offers comparable functionality: anonymous functions.
In Go, anonymous functions are declared using the func keyword followed by the function parameters and body. Unlike named functions, anonymous functions don't have a name and are typically defined and used inline within other code. Here's an example:
func main() { // Define an anonymous function that returns a string stringy := func() string { return "Stringy function" } // Pass the anonymous function as an argument to another function takesAFunction(stringy) } func takesAFunction(foo func() string) { fmt.Printf("takesAFunction: %v\n", foo()) }
In this example, we define an anonymous function named stringy that returns a string. We then pass stringy as an argument to the takesAFunction() function, which prints the result of calling stringy.
Anonymous functions can also be used to return functions. Here's an example:
func main() { // Define an anonymous function that returns a string stringy := func() string { return "bar" // have to return a string to be stringy } // Return the anonymous function as the result of another function returnsAFunction()() } func returnsAFunction() func() string { return func() string { fmt.Printf("Inner stringy function\n") return "bar" } }
In this example, the returnsAFunction() function returns an anonymous function that returns a string. We then call the returned anonymous function, which prints a message and returns a string.
The above is the detailed content of How Does Go Achieve Lambda Expression Functionality Without Explicit Support?. For more information, please follow other related articles on the PHP Chinese website!
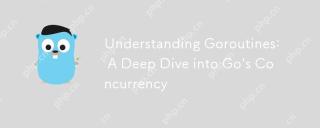
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
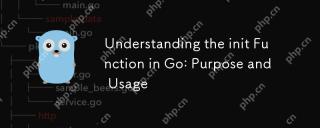
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
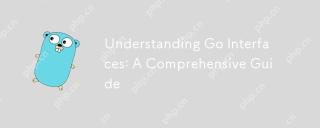
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
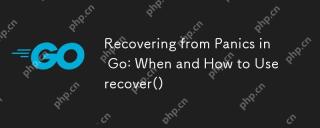
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
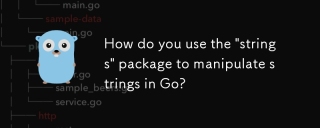
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
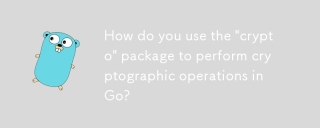
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
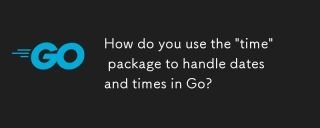
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
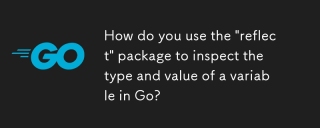
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
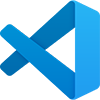
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
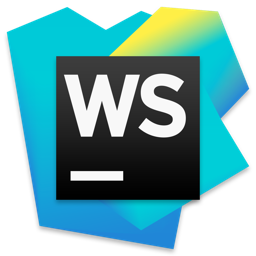
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
