In Express.js, middlewares are special functions that have access to the request (req), response (res), and a third parameter called next. Unlike regular route handlers, middlewares play a critical role in controlling the flow of the application by executing external logic before the main business logic.
How Do Middlewares Work?
When an HTTP request hits an Express.js server, it flows through a series of middleware functions. Each middleware can:
- Modify the request object (e.g., attach data, validate tokens).
- Modify the response object (e.g., send a response early).
- Pass control to the next middleware in the stack using the next() function.
If a middleware doesn't call next(), the request-response cycle terminates there, and no further logic (including route handlers) will execute.
Why Do We Use Middlewares?
Middlewares are perfect for scenarios where we need to add reusable logic before processing a request. For instance:
- Authentication: Checking if the user is logged in (e.g., validating - JWT tokens).
- Authorization: Ensuring users have the necessary permissions to perform certain actions (e.g., admins can delete content).
- Request Validation: Verifying if all required inputs are provided.
- Logging and Monitoring: Recording details of incoming requests for analytics or debugging.
- Error Handling: Catching errors globally to send meaningful responses.
Defining and Using Middlewares
A middleware function looks like this:
app.use((req, res, next) => { // Logic here next(); // Pass control to the next middleware or route handler });
- req (Request): Contains information about the incoming HTTP request (e.g., headers, body, params).
- res (Response): Used to send data back to the client. next(): A function that passes control to the next middleware in line.
Middleware Flow: Order of Execution
Middleware order matters! Express executes middlewares sequentially in the order they are defined.
If a middleware is defined after a route, it will not affect that route. This is why middlewares must be declared before routes in your app.js.
Example:
// Middleware to check if the user has admin privileges app.use((req, res, next) => { console.log("Checking for admin role..."); // Simulating a user object attached earlier in the pipeline if (req.user && req.user.role === "admin") { console.log("Access granted"); next(); // Move to the next middleware or route handler } else { console.log("Access denied"); res.status(403).send("You do not have access to this resource."); } }); // Routes app.get("/admin/dashboard", (req, res) => { res.send("Welcome to the admin dashboard!"); }); app.get("/public", (req, res) => { res.send("This is a public page."); });
Internal Execution Flow
Here’s what happens step by step:
- Incoming Request: A request hits the server.
-
Middleware Execution:
- The middleware checks the req.user.role.
- If role is "admin", it calls next() to pass control to the next middleware or route.
- If not, the middleware terminates the request by sending a 403 Forbidden response.
- Route Handler: If next() is called, the relevant route handler (e.g., /admin/dashboard) executes.
Example Flow:
- A user with role: "admin" requests /admin/dashboard.
- Middleware logs "Access granted" and calls next().
- Route handler sends "Welcome to the admin dashboard!".
- A user with role: "user" requests /admin/dashboard.
- Middleware logs "Access denied" and sends "You do not have access to this resource".
Key Takeaways
- Middlewares are like gatekeepers—they decide what happens before the main route logic runs.
- Use next() to ensure the flow continues to the next middleware or route.
- Always define critical middlewares before the routes to ensure they apply.
- If you don’t call next() or send a response, the request will hang (timed out).
The above is the detailed content of Understanding Middlewares in Express.js and Their Internal Working. For more information, please follow other related articles on the PHP Chinese website!
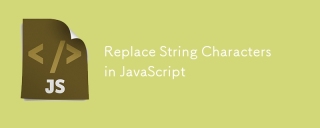
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
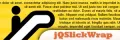
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
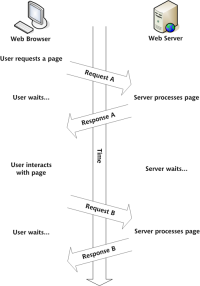
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
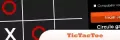
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
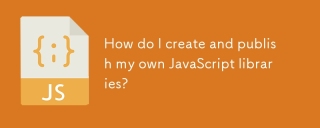
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
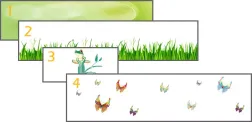
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
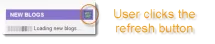
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery
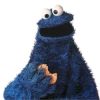
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
