


Iterating Over Overlapping Pairs of List Values
When iterating over a Python list, it's often necessary to access both the current and subsequent elements. While using the zip function to pair consecutive values is effective, there may be a more efficient approach.
Using the pairwise() Function
Python 3.8 provides the itertools.pairwise() function, which pairs successive elements of an iterable:
import itertools def pairwise(iterable): "s -> (s0, s1), (s1, s2), (s2, s3), ..." a, b = itertools.tee(iterable) next(b, None) return zip(a, b)
This function creates two iterators, a and b, pointing to the first element of the input iterable. b is advanced one step, resulting in a pointing to the current element and b pointing to the next element. zip is then used to pair the elements from both iterators.
Example Usage:
the_list = ['a', 'b', 'c', 'd'] for current, next in pairwise(the_list): print(current, next) # Output: # a b # b c # c d
Caveats:
It's crucial to note that pairwise() functions by iterating over the iterable multiple times. This means that if one iterator advances significantly faster than others, the implementation may retain consumed elements in memory to ensure they're available to all iterators.
Other Options for N-element Windows
The pairwise() function can be extended to create windows of arbitrary sizes:
def n_wise(iterable, n): "s -> (s0, s1, ..., s(n-1)), (s1, s2, ..., s(n)), ..." iterators = itertools.tee(iterable, n) for i in range(1, n): next(iterators[i], None) return zip(*iterators)
For example, to iterate over triples in a list:
for triplet in n_wise(the_list, 3): print(*triplet) # Output: # a b c # b c d
Conclusion:
While the traditional method of iterating over overlapping pairs using zip is viable, the pairwise() and n_wise functions offer a concise and efficient way to achieve the same result for windows of any size.
The above is the detailed content of How Can I Efficiently Iterate Over Overlapping Pairs (or N-element Windows) of List Values in Python?. For more information, please follow other related articles on the PHP Chinese website!
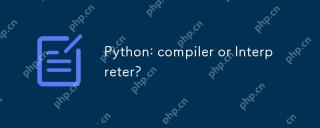
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
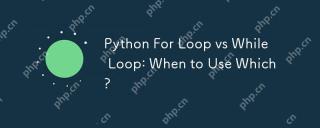
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
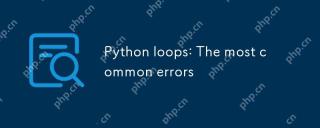
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
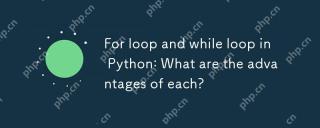
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
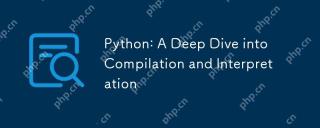
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
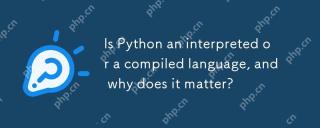
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
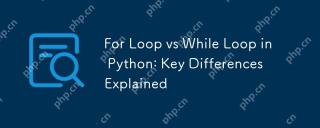
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
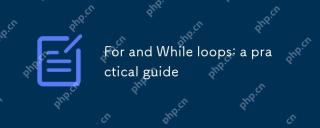
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
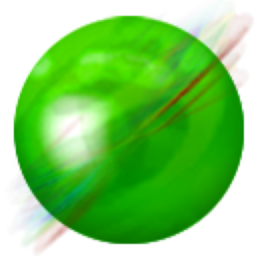
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
