


Implementing Route Guards in React: Protecting Your Routes with Authentication and Roles
Route Guards in React
Route Guards in React refer to the mechanism that restricts or allows access to certain routes based on specific conditions or criteria, such as user authentication, roles, permissions, or even data availability. This is a common requirement in applications with private or protected routes (e.g., admin dashboards, user profiles, or other sensitive areas).
Route guards prevent unauthorized users from accessing restricted routes by either redirecting them to another page or displaying an error message when they try to access a route they are not permitted to view.
In React, route guards can be implemented by utilizing React Router in combination with custom logic, such as authentication states or role-based access control.
How Route Guards Work
- Check User Authentication: Determine if the user is logged in.
- Redirect or Restrict Access: If the user is not authorized, either redirect them to a login page, a permission-denied page, or any other appropriate view.
- Access Control Based on Role: For apps with multiple user roles (e.g., admin, guest, or regular user), restrict access based on the user's role.
Example of Implementing Route Guards in React
Let’s look at how to implement route guards using React Router with a basic authentication guard. We'll check if the user is authenticated and conditionally render the routes based on that.
Basic Example: Protecting Routes Based on Authentication
In this example, we will use a route guard to check if the user is authenticated before allowing access to a restricted route like /dashboard.
Step 1: Create the Route Guard Component
We’ll create a PrivateRoute component that checks if a user is authenticated and either allows access to the protected route or redirects them to the login page.
import React from 'react'; import { Route, Navigate } from 'react-router-dom'; // PrivateRoute component for protecting routes const PrivateRoute = ({ element, isAuthenticated, ...rest }) => { return ( <route element="{isAuthenticated" : to="/login"></route>} /> ); }; export default PrivateRoute;
- The PrivateRoute component takes the element (which is the protected route component), the isAuthenticated boolean, and the rest of the route props (...rest).
- If isAuthenticated is true, it renders the protected component (element). If not, it redirects the user to the login page using the
component.
Step 2: Set Up Routes with Route Guards
Now, let’s set up the main application where we use the PrivateRoute to protect certain routes like /dashboard.
import React from 'react'; import { Route, Navigate } from 'react-router-dom'; // PrivateRoute component for protecting routes const PrivateRoute = ({ element, isAuthenticated, ...rest }) => { return ( <route element="{isAuthenticated" : to="/login"></route>} /> ); }; export default PrivateRoute;
Explanation:
- We use a simple isAuthenticated state to track whether the user is logged in or not.
- If the user is logged in (i.e., isAuthenticated is true), they can access the /dashboard route. Otherwise, they are redirected to the /login page.
- The PrivateRoute component is used to guard the /dashboard route.
- We toggle the isAuthenticated state using the login button. If the user clicks the button, they are either logged in or logged out.
Example 2: Route Guards Based on User Roles
In this example, we'll implement a route guard that only allows access to the /admin route if the user has an admin role.
Step 1: Create a Role-Based Route Guard
We will modify the PrivateRoute component to handle both authentication and role-based access.
import React, { useState } from 'react'; import { BrowserRouter, Routes, Route, Link } from 'react-router-dom'; import PrivateRoute from './PrivateRoute'; // Import the route guard // Simple page components const Home = () => <h2 id="Home-Page">Home Page</h2>; const Login = () => <h2 id="Login-Page">Login Page</h2>; const Dashboard = () => <h2 id="Dashboard-Private">Dashboard (Private)</h2>; const App = () => { const [isAuthenticated, setIsAuthenticated] = useState(false); // Authentication state return ( <browserrouter> <nav> <ul> <li> <link to="/">Home</li> <li> <link to="/login">Login</li> <li> <link to="/dashboard">Dashboard</li> </ul> </nav> <routes> <route path="/" element="{<Home"></route>} /> <route path="/login" element="{<Login"></route>} /> {/* Protected route using PrivateRoute */} <privateroute path="/dashboard" isauthenticated="{isAuthenticated}" element="{<Dashboard"></privateroute>} /> </routes> <div> {/* Toggle authentication state */} <button onclick="{()"> setIsAuthenticated(!isAuthenticated)}> {isAuthenticated ? "Logout" : "Login"} </button> </div> </browserrouter> ); }; export default App;
Step 2: Protect Routes Based on Role
In the main application, we’ll set up a role-based route guard that only allows access to the /admin route if the user is an admin.
import React from 'react'; import { Route, Navigate } from 'react-router-dom'; // Role-based Route Guard const RoleBasedRoute = ({ element, isAuthenticated, userRole, requiredRole, ...rest }) => { return ( <route element="{" isauthenticated userrole="==" requiredrole : to="/login"></route> } /> ); }; export default RoleBasedRoute;
Explanation:
- The RoleBasedRoute component checks both if the user is authenticated and whether their role matches the required role for the route (e.g., only users with the role 'admin' can access the /admin page).
- The userRole state determines if the user is an admin or a regular user.
- You can toggle between 'user' and 'admin' roles using the button.
Conclusion
Route guards are an essential feature for controlling access to specific parts of your application based on conditions like authentication and user roles. They help improve the security and functionality of your app by ensuring that only authorized users can access certain routes. React Router makes it easy to implement route guards by using conditional rendering, the Navigate component for redirection, and custom components to handle complex logic.
The above is the detailed content of Implementing Route Guards in React: Protecting Your Routes with Authentication and Roles. For more information, please follow other related articles on the PHP Chinese website!
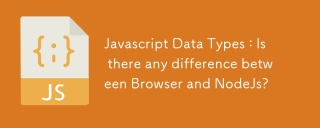
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
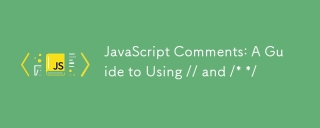
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
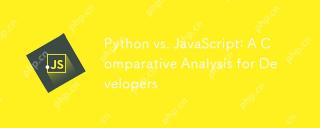
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
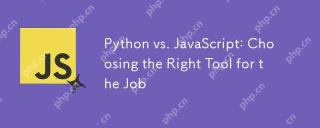
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
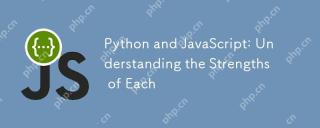
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
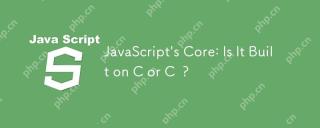
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
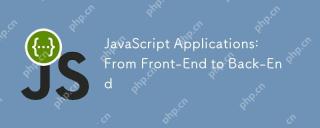
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
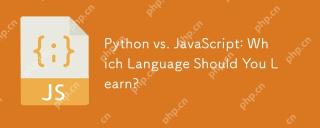
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
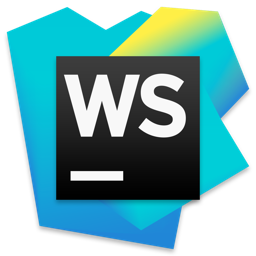
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
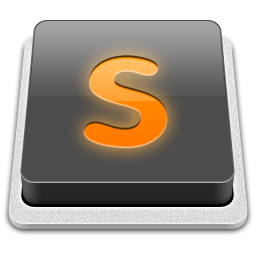
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
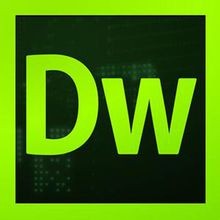
Dreamweaver CS6
Visual web development tools
