Builder Pattern and Its Applications
The Builder Pattern offers a solution to the challenge of creating objects with numerous construction parameters. Consider a scenario where you encounter constructors with multiple parameters, making it cumbersome to determine their order and appropriate configuration.
Common Use Cases
- Complex Object Construction: The Builder Pattern allows you to construct objects in a step-by-step manner using setters or "build" methods that apply logic and validation.
- Immutable Objects: Using a builder allows for immutable objects, ensuring that once constructed, the object's state cannot be modified.
- Extensibility: The Builder Pattern makes it easy to add new construction parameters to your objects without the need to modify the existing constructor.
Advantages over Factory Pattern
While Factory Patterns are useful for creating objects from a single factory method, they offer less control over the creation process. With the Builder Pattern:
- You can incrementally build objects, allowing for more complex configurations.
- It promotes code clarity by consolidating parameter configuration in one location.
- It supports chaining of method calls for fluent construction.
Example in Java
Consider a Pizza class with parameters for size, cheese, pepperoni, and bacon.
public class PizzaBuilder { private int size; private boolean cheese; private boolean pepperoni; private boolean bacon; public PizzaBuilder(int size) { this.size = size; } public PizzaBuilder cheese(boolean value) { cheese = value; return this; } public PizzaBuilder pepperoni(boolean value) { pepperoni = value; return this; } public PizzaBuilder bacon(boolean value) { bacon = value; return this; } public Pizza build() { return new Pizza(this); } } public class Pizza { private final int size; private final boolean cheese; private final boolean pepperoni; private final boolean bacon; private Pizza(PizzaBuilder builder) { this.size = builder.size; this.cheese = builder.cheese; this.pepperoni = builder.pepperoni; this.bacon = builder.bacon; } }
With this builder, constructing a pizza becomes intuitive:
Pizza pizza = new PizzaBuilder(12) .cheese(true) .pepperoni(true) .bacon(true) .build();
The Builder Pattern provides a flexible and efficient solution for constructing complex objects with numerous parameters. It enhances code readability, extensibility, and the ability to handle object creation with customizable options.
The above is the detailed content of How Does the Builder Pattern Solve Complex Object Construction Challenges?. For more information, please follow other related articles on the PHP Chinese website!
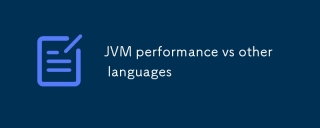
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
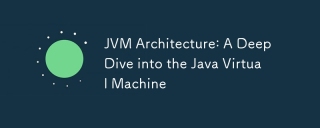
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
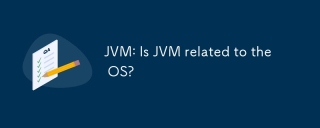
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
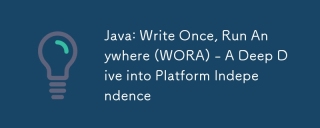
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
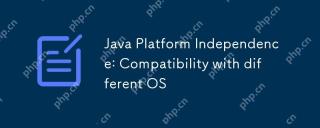
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
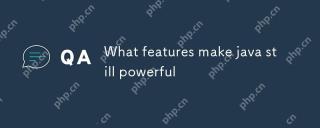
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
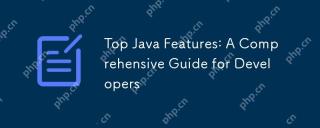
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
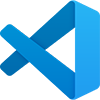
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
