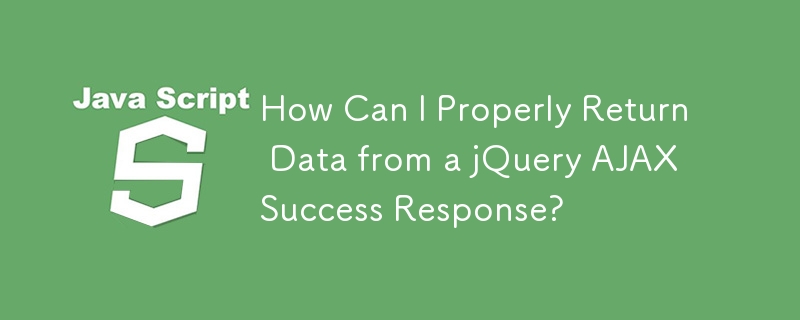
Return Data from jQuery AJAX Success Response
Problem Overview:
You're attempting to return data from a successful jQuery AJAX call, but encountering issues.
Incorrect Approaches:
- Returning data directly from the success callback: This won't work because the function is asynchronous.
- Returning data from the function outside the success callback: This won't work either because the data is not immediately available.
Solution: Using Promises
Promises provide a way to handle asynchronous operations. Here's how to return data using promises:
function testAjax() {
return $.ajax({
url: "getvalue.php",
});
}
// Get promise from the testAjax function
var promise = testAjax();
// Once the data is available, handle it in the then block
promise.then(function (data) {
alert(data); // Use the data here
});
Simplified Syntax with Promises/A :
Current versions of jQuery (3.x and above) support Promises/A , which allows for simplified syntax:
testAjax()
.then(data => alert(data));
.catch(error => alert(error)); // Handle errors here
Benefits of Promises:
-
Asynchronous handling: Promises allow you to work with the data when it becomes available, not immediately after the AJAX call.
-
Chaining: Promises can be chained together to perform multiple asynchronous tasks in sequence.
-
Exception handling: Promises support exception handling through the catch method.
Additional Notes:
- If you need to return a real Promise (instead of a jQuery Deferred object), you can use a Promise polyfill or convert the jQuery promise using the methods described in the linked resources below.
- Always handle rejections (errors) in your promise chains to avoid unhandled exceptions.
- Check browser compatibility and use polyfills or transpilers as needed for support in older browsers.
The above is the detailed content of How Can I Properly Return Data from a jQuery AJAX Success Response?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn