


How to Efficiently Access and Modify Nested Dictionary Items Using a Key List in Python?
Accessing Nested Dictionary Items with a Key List
When dealing with complex dictionary structures, traversing them to access specific items can be a common challenge. Finding an efficient and flexible approach to navigate these dictionaries is crucial.
Consider the scenario where you have a nested dictionary like:
dataDict = { "a": { "r": 1, "s": 2, "t": 3 }, "b": { "u": 1, "v": { "x": 1, "y": 2, "z": 3 }, "w": 3 } }
You may need to access these items using a list of keys, such as ["a", "r"] or ["b", "v", "y"]. While the approach below may work:
# Get a given data from a dictionary with position provided as a list def getFromDict(dataDict, mapList): for k in mapList: dataDict = dataDict[k] return dataDict
There exists a more efficient method using Python's reduce():
from functools import reduce # forward compatibility for Python 3 import operator def getFromDict(dataDict, mapList): return reduce(operator.getitem, mapList, dataDict)
This improved method utilizes reduce to traverse the dictionary, using the operators.getitem function to access each nested item using the key list.
For setting values, we can leverage getFromDict to locate the correct 'parent' dictionary and then assign the value:
def setInDict(dataDict, mapList, value): getFromDict(dataDict, mapList[:-1])[mapList[-1]] = value
This method efficiently traverses the dictionary to the appropriate location in the data structure and updates the value.
Finally, we can provide snake_case function names and extend these functions to work with mixed data types, resulting in:
from functools import reduce # forward compatibility for Python 3 import operator def get_by_path(root, items): return reduce(operator.getitem, items, root) def set_by_path(root, items, value): get_by_path(root, items[:-1])[items[-1]] = value def del_by_path(root, items): del get_by_path(root, items[:-1])[items[-1]]
These functions provide a versatile utility for navigating and manipulating nested dictionaries, lists, or a mix of the two.
The above is the detailed content of How to Efficiently Access and Modify Nested Dictionary Items Using a Key List in Python?. For more information, please follow other related articles on the PHP Chinese website!

ToappendelementstoaPythonlist,usetheappend()methodforsingleelements,extend()formultipleelements,andinsert()forspecificpositions.1)Useappend()foraddingoneelementattheend.2)Useextend()toaddmultipleelementsefficiently.3)Useinsert()toaddanelementataspeci
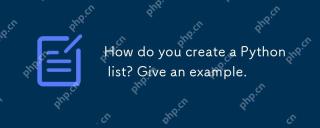
TocreateaPythonlist,usesquarebrackets[]andseparateitemswithcommas.1)Listsaredynamicandcanholdmixeddatatypes.2)Useappend(),remove(),andslicingformanipulation.3)Listcomprehensionsareefficientforcreatinglists.4)Becautiouswithlistreferences;usecopy()orsl
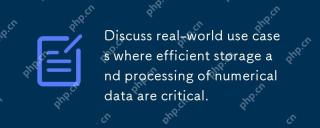
In the fields of finance, scientific research, medical care and AI, it is crucial to efficiently store and process numerical data. 1) In finance, using memory mapped files and NumPy libraries can significantly improve data processing speed. 2) In the field of scientific research, HDF5 files are optimized for data storage and retrieval. 3) In medical care, database optimization technologies such as indexing and partitioning improve data query performance. 4) In AI, data sharding and distributed training accelerate model training. System performance and scalability can be significantly improved by choosing the right tools and technologies and weighing trade-offs between storage and processing speeds.
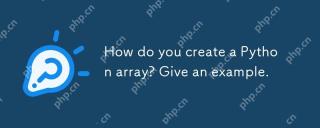
Pythonarraysarecreatedusingthearraymodule,notbuilt-inlikelists.1)Importthearraymodule.2)Specifythetypecode,e.g.,'i'forintegers.3)Initializewithvalues.Arraysofferbettermemoryefficiencyforhomogeneousdatabutlessflexibilitythanlists.
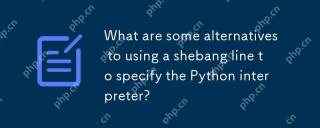
In addition to the shebang line, there are many ways to specify a Python interpreter: 1. Use python commands directly from the command line; 2. Use batch files or shell scripts; 3. Use build tools such as Make or CMake; 4. Use task runners such as Invoke. Each method has its advantages and disadvantages, and it is important to choose the method that suits the needs of the project.
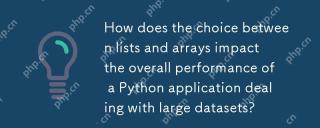
ForhandlinglargedatasetsinPython,useNumPyarraysforbetterperformance.1)NumPyarraysarememory-efficientandfasterfornumericaloperations.2)Avoidunnecessarytypeconversions.3)Leveragevectorizationforreducedtimecomplexity.4)Managememoryusagewithefficientdata
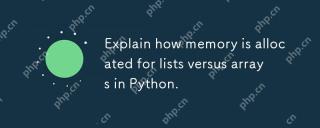
InPython,listsusedynamicmemoryallocationwithover-allocation,whileNumPyarraysallocatefixedmemory.1)Listsallocatemorememorythanneededinitially,resizingwhennecessary.2)NumPyarraysallocateexactmemoryforelements,offeringpredictableusagebutlessflexibility.
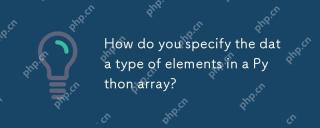
InPython, YouCansSpectHedatatYPeyFeLeMeReModelerErnSpAnT.1) UsenPyNeRnRump.1) UsenPyNeRp.DLOATP.PLOATM64, Formor PrecisconTrolatatypes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
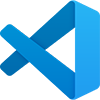
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
