


How to Implement Access Control Lists (ACLs) and Role-Based Access in a Web MVC Application?
How can I implement an Access Control List in my Web MVC application and how to handle user role-based access?
ACL Implementation
The decorator pattern is an effective way to implement ACLs without extending the Controller class. Here's how:
class SecureContainer { protected $target; protected $acl; public function __construct($target, $acl) { $this->target = $target; $this->acl = $acl; } public function __call($method, $arguments) { if (method_exists($this->target, $method) && $this->acl->isAllowed(get_class($this->target), $method)) { return call_user_func_array([$this->target, $method], $arguments); } } }
You can use this as follows:
$currentUser = ...; $controller = ...; $acl = new AccessControlList($currentUser); $controller = new SecureContainer($controller, $acl); $controller->actionIndex(); // ACL-protected controller methods
User Role-Based Access
For role-based access, consider the following:
Checking Owner of a Resource:
- Pass the object itself to the ACL for permission checks.
- If the object lacks the necessary details, provide them explicitly.
For example:
$this->acl->isAllowed( $this->target->getPermissions(), // Get object permissions [$getter, $method] // Command );
Enforcing Access Restrictions:
- Consider using a service layer to abstract object access and ACL checks.
- The service can interact with domain objects to gather necessary details.
Additional Notes on MVC:
- Model refers to a layer, not a specific class.
- Domain Business Logic handles calculations and conditions without concern for data storage.
- Data Access and Storage manages SQL statements or data retrieval mechanisms.
- Services provide abstraction and facilitation for reusable components.
The above is the detailed content of How to Implement Access Control Lists (ACLs) and Role-Based Access in a Web MVC Application?. For more information, please follow other related articles on the PHP Chinese website!
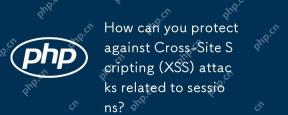
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
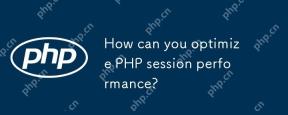
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
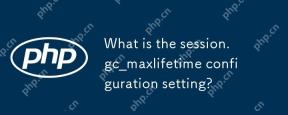
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
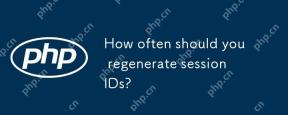
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
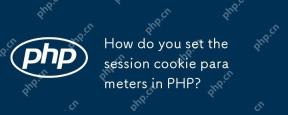
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
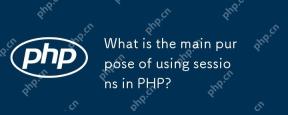
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
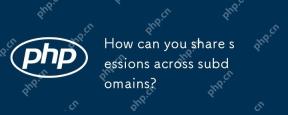
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!
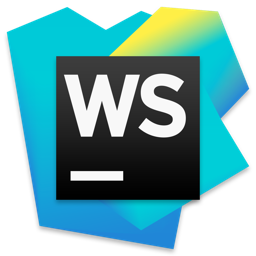
WebStorm Mac version
Useful JavaScript development tools
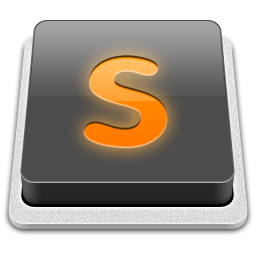
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version