


Merging and Decoding Bytes into Boolean Values
In some programming scenarios, it becomes necessary to manipulate binary data at a bit level. One such task involves converting eight boolean values into a single byte and vice versa. This article explores efficient methods to accomplish this conversion.
Converting Boolean Values to Byte
The simplest approach to merging boolean values into a byte is through bitwise operations. Each boolean value corresponds to a bit position in the byte, with 'true' assigned to '1' and 'false' to '0'. By iteratively shifting '1' left based on the boolean value and ORing it with the accumulating result, a byte can be constructed. This process is exemplified in the following function:
unsigned char ToByte(bool b[8]) { unsigned char c = 0; for (int i=0; i <h3 id="Decoding-a-Byte-into-Boolean-Values">Decoding a Byte into Boolean Values</h3><p>Conversely, decoding a byte into individual boolean values requires a similar iterative process. By shifting the byte right and comparing it against a '1' mask, each bit position can be extracted and assigned a boolean value of 'true' for '1' and 'false' for '0'. The following function implements this process:</p><pre class="brush:php;toolbar:false">void FromByte(unsigned char c, bool b[8]) { for (int i=0; i <h3 id="Bitfield-Approach">Bitfield Approach</h3><p>An alternative and potentially more elegant approach utilizes bitfields and unions. By defining a structure with eight 1-bit fields, each boolean value can be assigned directly to the corresponding field. Unioning this structure with an unsigned char allows effortless conversion between the two data types. This is achieved through the following code snippet:</p><pre class="brush:php;toolbar:false">struct Bits { unsigned b0:1, b1:1, b2:1, b3:1, b4:1, b5:1, b6:1, b7:1; }; union CBits { Bits bits; unsigned char byte; };
By assigning values to either member of the union, the conversion is automatically performed. However, it is crucial to note that bitfield order and potential padding may vary depending on the implementation.
The above is the detailed content of How Can I Efficiently Convert Between Eight Boolean Values and a Single Byte?. For more information, please follow other related articles on the PHP Chinese website!
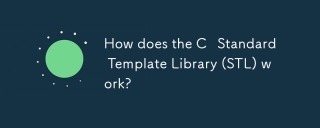
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
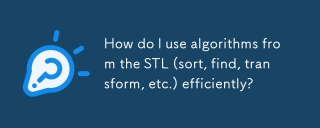
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
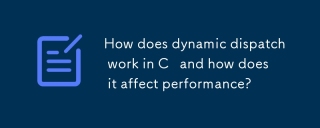
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
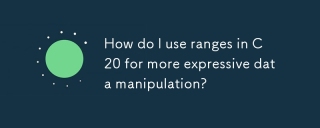
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
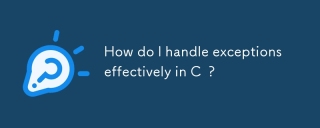
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
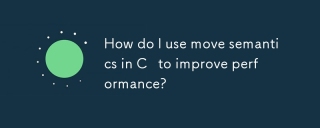
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
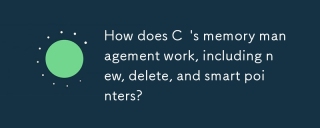
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
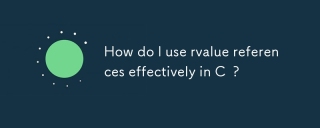
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
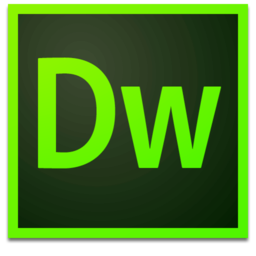
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
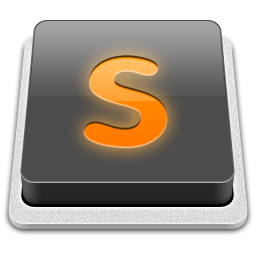
SublimeText3 Mac version
God-level code editing software (SublimeText3)
