Parsing Comma-separated Strings into Integer Arrays
In C , parsing a comma-separated string into an integer array can be achieved using the following steps:
- Read one number at a time: Use an input stream (std::istringstream) to read numbers from the string character by character.
- Check for commas: After reading a number, check if the next character is a comma (','). If so, ignore it.
- Push numbers into an array: Iterate over the input stream until all numbers are extracted. Store each number in a dynamically growing array (e.g., std::vector).
Here's an example code snippet that demonstrates this approach:
#include <vector> #include <string> #include <sstream> #include <iostream> int main() { std::string str = "1,2,3,4,5,6"; std::vector<int> vect; std::stringstream ss(str); for (int i; ss >> i;) { vect.push_back(i); if (ss.peek() == ',') ss.ignore(); } for (std::size_t i = 0; i <p>Output:</p> <pre class="brush:php;toolbar:false">1 2 3 4 5 6
The above is the detailed content of How to Parse a Comma-Separated String into an Integer Array in C ?. For more information, please follow other related articles on the PHP Chinese website!
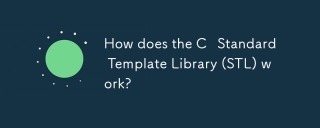
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
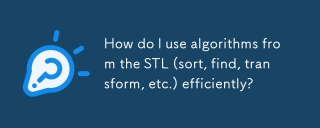
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
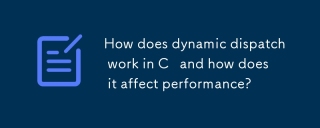
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
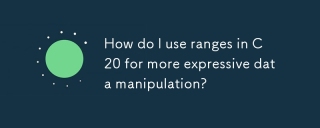
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
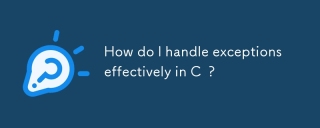
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
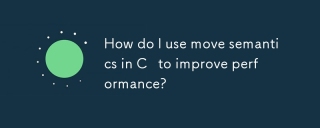
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
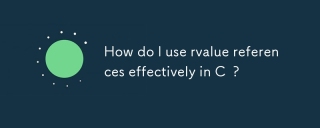
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
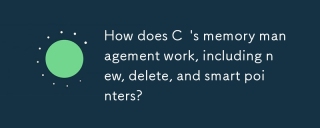
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
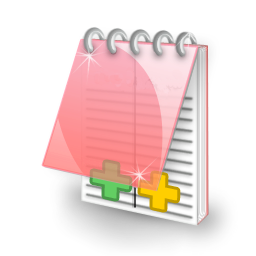
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
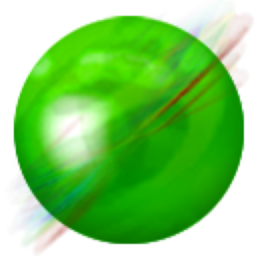
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
