Multithreading in Python: A Simplified Approach
Threading is a technique used to divide tasks across multiple threads, improving the efficiency of a program.
Simplified Example Using Map and Pool
In Python, multithreading has been greatly simplified with the introduction of map and pool. Here's a concise example:
from multiprocessing.dummy import Pool as ThreadPool pool = ThreadPool(4) results = pool.map(my_function, my_array)
This code snippet effectively distributes the execution of my_function across 4 available threads. The resulting values are stored in the results list.
Map Function: A Functional Abstraction
The map function, inherited from functional languages like Lisp, iterates over a sequence, applies a function to each element, and collects the results into a list. It abstracts the iteration process, making multithreading effortless.
Thread Pool: Managing Threads
In the code above, the ThreadPool creates a pool of 4 worker threads. These threads execute the tasks assigned by the map function. Once all tasks are complete, the pool closes, ensuring that all threads finish their operations.
Implementation Notes
- Use multiprocessing.Pool for CPU-intensive tasks, and multiprocessing.dummy.Pool for I/O-related tasks.
- For passing multiple arguments to a thread, use starmap with zip to combine arrays, or with itertools.repeat to pass a constant and an array.
The above is the detailed content of How Can Python's `map` and `Pool` Simplify Multithreading?. For more information, please follow other related articles on the PHP Chinese website!
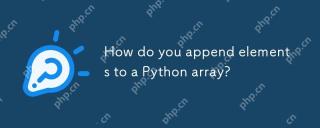
InPython,youappendelementstoalistusingtheappend()method.1)Useappend()forsingleelements:my_list.append(4).2)Useextend()or =formultipleelements:my_list.extend(another_list)ormy_list =[4,5,6].3)Useinsert()forspecificpositions:my_list.insert(1,5).Beaware

The methods to debug the shebang problem include: 1. Check the shebang line to make sure it is the first line of the script and there are no prefixed spaces; 2. Verify whether the interpreter path is correct; 3. Call the interpreter directly to run the script to isolate the shebang problem; 4. Use strace or trusts to track the system calls; 5. Check the impact of environment variables on shebang.
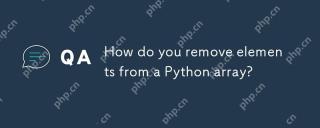
Pythonlistscanbemanipulatedusingseveralmethodstoremoveelements:1)Theremove()methodremovesthefirstoccurrenceofaspecifiedvalue.2)Thepop()methodremovesandreturnsanelementatagivenindex.3)Thedelstatementcanremoveanitemorslicebyindex.4)Listcomprehensionscr
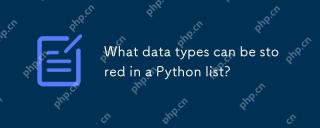
Pythonlistscanstoreanydatatype,includingintegers,strings,floats,booleans,otherlists,anddictionaries.Thisversatilityallowsformixed-typelists,whichcanbemanagedeffectivelyusingtypechecks,typehints,andspecializedlibrarieslikenumpyforperformance.Documenti
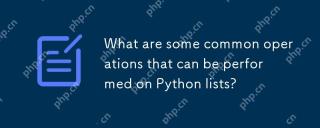
Pythonlistssupportnumerousoperations:1)Addingelementswithappend(),extend(),andinsert().2)Removingitemsusingremove(),pop(),andclear().3)Accessingandmodifyingwithindexingandslicing.4)Searchingandsortingwithindex(),sort(),andreverse().5)Advancedoperatio
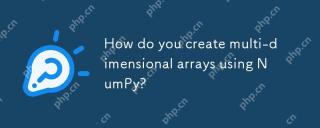
Create multi-dimensional arrays with NumPy can be achieved through the following steps: 1) Use the numpy.array() function to create an array, such as np.array([[1,2,3],[4,5,6]]) to create a 2D array; 2) Use np.zeros(), np.ones(), np.random.random() and other functions to create an array filled with specific values; 3) Understand the shape and size properties of the array to ensure that the length of the sub-array is consistent and avoid errors; 4) Use the np.reshape() function to change the shape of the array; 5) Pay attention to memory usage to ensure that the code is clear and efficient.
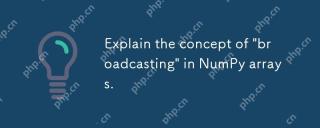
BroadcastinginNumPyisamethodtoperformoperationsonarraysofdifferentshapesbyautomaticallyaligningthem.Itsimplifiescode,enhancesreadability,andboostsperformance.Here'showitworks:1)Smallerarraysarepaddedwithonestomatchdimensions.2)Compatibledimensionsare
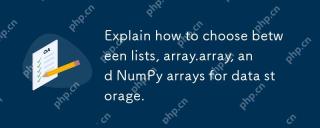
ForPythondatastorage,chooselistsforflexibilitywithmixeddatatypes,array.arrayformemory-efficienthomogeneousnumericaldata,andNumPyarraysforadvancednumericalcomputing.Listsareversatilebutlessefficientforlargenumericaldatasets;array.arrayoffersamiddlegro


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
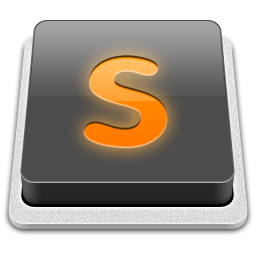
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
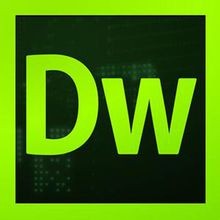
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
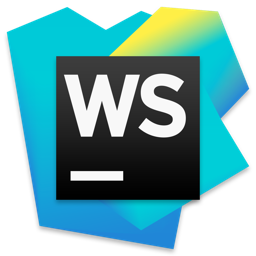
WebStorm Mac version
Useful JavaScript development tools
