Converting List
Java programmers often encounter the need to convert a List
1. Using mapToInt()
Streams provide the mapToInt() method, which allows you to convert each element of a stream to a primitive int value. To convert a List
import java.util.List; class ListToIntArrayExample { public static void main(String[] args) { List<integer> list = List.of(1, 2, 3, 4, 5); // Convert the list to an int[] using mapToInt() int[] intArray = list.stream() .mapToInt(Integer::intValue) .toArray(); // Print the converted int[] for (int i : intArray) { System.out.println(i); } } }</integer>
In this example, we use the stream() method on the list to create a stream of integers. We then apply the mapToInt() method with a lambda expression that simply returns the integer value of each element. Finally, we use toArray() to convert the stream back to an int[].
2. Using Unboxing
Since the compiler knows that the result of the lambda in mapToInt() must be of type int, we can simplify the lambda by omitting the explicit cast to int:
... int[] intArray = list.stream() .mapToInt(i -> i) .toArray(); ...
3. Inferring the Generic Type
Since the compiler can infer the generic type of the stream, we can omit the Integer type in the lambda:
... int[] intArray = list.stream() .mapToInt(i -> i) .toArray(); ...
These methods provide efficient and straightforward ways to convert a List
The above is the detailed content of How Can I Efficiently Convert a List to an int[] in Java?. For more information, please follow other related articles on the PHP Chinese website!
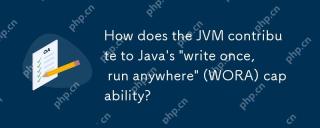
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
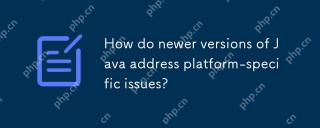
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
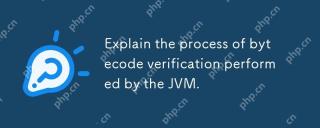
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.
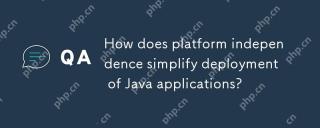
Java'splatformindependenceallowsapplicationstorunonanyoperatingsystemwithaJVM.1)Singlecodebase:writeandcompileonceforallplatforms.2)Easyupdates:updatebytecodeforsimultaneousdeployment.3)Testingefficiency:testononeplatformforuniversalbehavior.4)Scalab
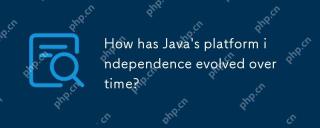
Java's platform independence is continuously enhanced through technologies such as JVM, JIT compilation, standardization, generics, lambda expressions and ProjectPanama. Since the 1990s, Java has evolved from basic JVM to high-performance modern JVM, ensuring consistency and efficiency of code across different platforms.
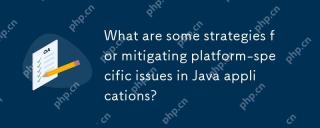
How does Java alleviate platform-specific problems? Java implements platform-independent through JVM and standard libraries. 1) Use bytecode and JVM to abstract the operating system differences; 2) The standard library provides cross-platform APIs, such as Paths class processing file paths, and Charset class processing character encoding; 3) Use configuration files and multi-platform testing in actual projects for optimization and debugging.
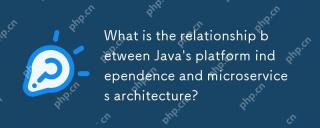
Java'splatformindependenceenhancesmicroservicesarchitecturebyofferingdeploymentflexibility,consistency,scalability,andportability.1)DeploymentflexibilityallowsmicroservicestorunonanyplatformwithaJVM.2)Consistencyacrossservicessimplifiesdevelopmentand
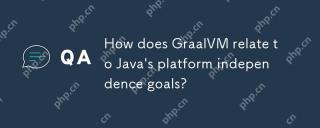
GraalVM enhances Java's platform independence in three ways: 1. Cross-language interoperability, allowing Java to seamlessly interoperate with other languages; 2. Independent runtime environment, compile Java programs into local executable files through GraalVMNativeImage; 3. Performance optimization, Graal compiler generates efficient machine code to improve the performance and consistency of Java programs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
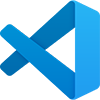
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
