Modifying Custom Annotation Values at Runtime
This question addresses the challenge of modifying annotation string parameters for a compiled class that exists within the classpath at runtime. Specifically, the goal is to alter the value of a parameter without affecting the original source code.
Solution
To address this challenge, we leverage Java's reflection capabilities and a custom Java agent.
- Java Reflection: Using reflection, we can access the annotation values at runtime. Specifically, we use the Proxy.getInvocationHandler method to obtain the handler of the annotation instance, and then the handler.getClass() and memberValues fields to access the actual values.
- Custom Java Agent: To modify the annotation value, a custom Java agent is required. This agent dynamically attaches to the running JVM, allowing us to intercept class loading, including the annotated class, and modify the annotation value during the load process.
Implementation
import java.lang.annotation.Annotation; import java.lang.instrument.Instrumentation; import java.lang.reflect.Field; import java.lang.reflect.InvocationHandler; import java.lang.reflect.Proxy; import java.util.Map; public class AnnotationModifierAgent { public static void premain(String agentArgs, Instrumentation inst) { inst.addTransformer((loader, className, classBeingRedefined, protectionDomain, classfileBuffer) -> { if (className.equals("your.package.Foobar")) { Class> foobarClass = loader.loadClass(className); Annotation[] annotations = foobarClass.getAnnotations(); for (Annotation annotation : annotations) { try { modifyAnnotationValue(annotation, "someProperty", "new value"); } catch (NoSuchFieldException | IllegalAccessException e) { e.printStackTrace(); } } } return classfileBuffer; }); } @SuppressWarnings("unchecked") public static void modifyAnnotationValue(Annotation annotation, String key, Object newValue) throws NoSuchFieldException, IllegalAccessException { InvocationHandler handler = Proxy.getInvocationHandler(annotation); Field f = handler.getClass().getDeclaredField("memberValues"); f.setAccessible(true); Map<string object> memberValues = (Map<string object>) f.get(handler); memberValues.put(key, newValue); } }</string></string>
Usage
To use this approach, you need to attach the custom Java agent to the JVM when it starts up. This can be achieved using the -javaagent option when launching the JVM.
java -javaagent:/path/to/AnnotationModifierAgent.jar your.main.class ...
Now, when the annotated class is loaded at runtime, the Java agent will intercept it and modify the annotation value accordingly.
The above is the detailed content of How Can I Modify Custom Annotation Values at Runtime in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
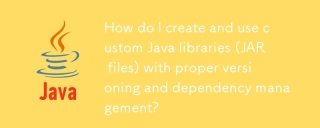
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
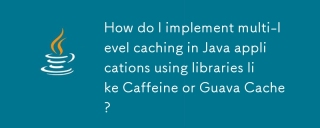
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
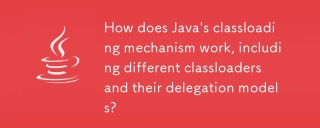
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
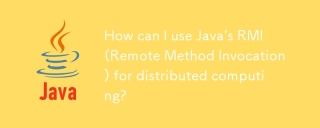
This article explains Java's Remote Method Invocation (RMI) for building distributed applications. It details interface definition, implementation, registry setup, and client-side invocation, addressing challenges like network issues and security.
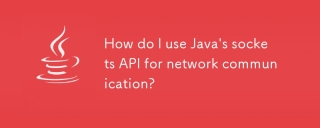
This article details Java's socket API for network communication, covering client-server setup, data handling, and crucial considerations like resource management, error handling, and security. It also explores performance optimization techniques, i
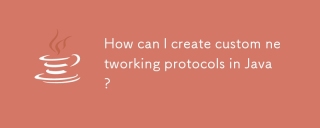
This article details creating custom Java networking protocols. It covers protocol definition (data structure, framing, error handling, versioning), implementation (using sockets), data serialization, and best practices (efficiency, security, mainta


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor
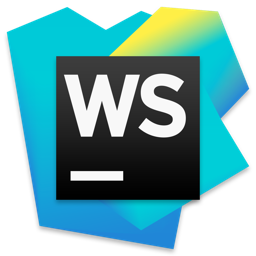
WebStorm Mac version
Useful JavaScript development tools
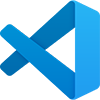
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.