


Local Variables in Nested Functions
Nested functions provide a convenient means of encapsulating specific functionality within a parent function. However, their closure behavior can introduce some complications in regard to the accessibility and value of local variables.
Problem:
Consider the following code snippet:
from functools import partial class Cage(object): def __init__(self, animal): self.animal = animal def gotimes(do_the_petting): do_the_petting() def get_petters(): for animal in ['cow', 'dog', 'cat']: cage = Cage(animal) def pet_function(): print("Mary pets the " + cage.animal + ".") yield (animal, partial(gotimes, pet_function)) funs = list(get_petters()) for name, f in funs: print(name + ":", f())
The desired behavior is to print three different animals ('cow', 'dog', 'cat') for each iteration. However, the program only prints 'cat' for all iterations. This behavior contradicts the expectation that the local variable cage is associated with the nested function.
Answer:
The misunderstanding lies in the assumption that a nested function stores a reference to its parent scope's local variables when it is defined. In reality, the nested function looks up variables from the parent scope only when it is executed.
In this specific example, the closure created for the pet_function indexes the cage variable from the get_petters function. When the pet_function is called, it accesses the closure to retrieve the value of cage. However, at that point, the get_petters function has completed, and the cage variable has a final value of 'cat'. Therefore, all subsequent calls to any pet_function variation return the value 'cat'.
Workarounds:
To resolve this issue, one can use various techniques to ensure that the nested function accesses the correct value of cage:
- Partial function: Utilize functools.partial to create a new function with a fixed value for cage.
- Scoped function: Create a new function within the loop to ensure a unique scope for each instance of pet_function.
- Default parameter value: Pass cage as a default parameter value to pet_function.
By employing one of these approaches, you can ensure that the nested function accesses the intended local variable for each iteration.
The above is the detailed content of Why Does My Nested Function Only Access the Final Value of a Local Variable in Its Parent Function?. For more information, please follow other related articles on the PHP Chinese website!
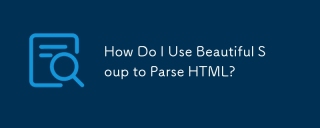
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
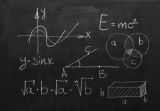
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
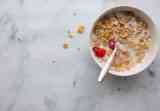
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
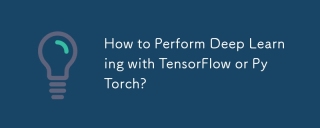
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
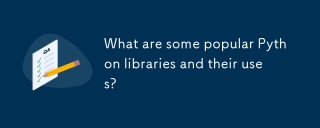
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
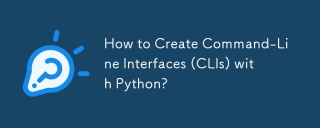
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
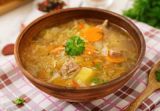
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
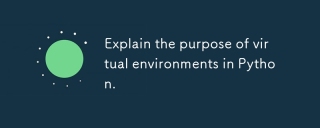
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
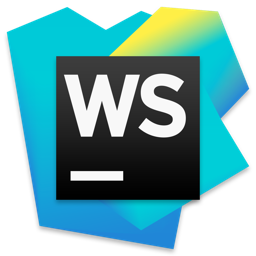
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
