


Why Copying an Empty Slice with copy() Results in an Empty Slice
When working with slices in Go, the copy() function is often used to create a copy of an existing slice. However, if the destination slice is empty, the copy operation behaves unexpectedly.
According to the documentation for copy():
The copy built-in function copies elements from a source slice into a destination slice. (As a special case, it also will copy bytes from a string to a slice of bytes.) The source and destination may overlap. Copy returns the number of elements copied, which will be the minimum of len(src) and len(dst).
This suggests that the number of elements copied should be the minimum of the lengths of the source and destination slices. However, when the destination slice is empty (as in your example where tmp is initialized as an empty slice), no elements are copied.
The reason for this behavior lies in the implementation of copy(). The function iterates over the source slice and copies elements to the destination slice until it reaches the end of either the source or destination slice. If the destination slice is empty, the loop will end immediately since there are no elements to copy.
To create a non-empty copy of a slice, the destination slice must be initialized with a non-zero length. This can be achieved using the make() function, as shown in the following example:
arr := []int{1, 2, 3} tmp := make([]int, len(arr)) copy(tmp, arr) fmt.Println(tmp) // Output: [1 2 3]
By explicitly setting the length of the destination slice, we ensure that copy() has enough space to copy all the elements from the source slice. This behavior is also documented in the Go Language Specification, which states that the number of elements copied is the minimum of the lengths of the source and destination slices.
Therefore, when attempting to copy an empty slice using copy(), it is important to initialize the destination slice with a non-zero length to ensure that the copy operation succeeds.
The above is the detailed content of Why Does `copy()` Return an Empty Slice When Copying to an Empty Destination Slice in Go?. For more information, please follow other related articles on the PHP Chinese website!
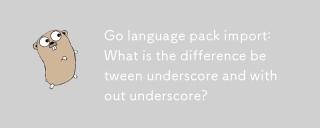
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
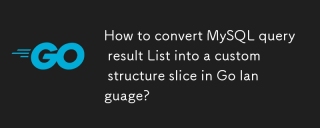
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
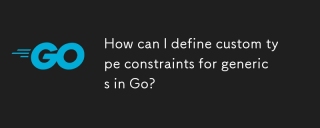
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
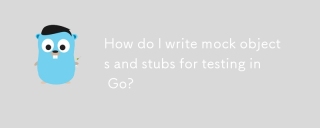
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
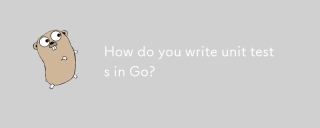
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
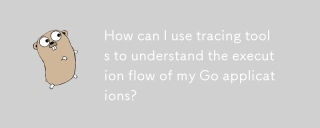
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
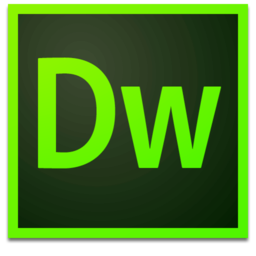
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
