JSON and Data Manipulation
JSON (JavaScript Object Notation) is a lightweight data format used for exchanging data between a server and a web application. It is widely supported across different programming languages and is a key component in modern web development.
Key Features of JSON:
1.Structure:
- Data is stored as key-value pairs in an object or as an array of values.
- Example (Object):
{ "name": "Damilare", "age": 30, "isEmployed": true, "hobbies": ["Singing", "Reading", "Coding"] }
- Example (Array):
["Dee", "Fred", "Inioluwa", "Iteoluwa"]
2.Data Exchange:
- JSON is often used to send and receive data between a server and a client.
Converting Between JSON and JavaScript
- Convert JavaScript to JSON Use JSON.stringify() to convert a JavaScript object or array into a JSON string.
Example: JavaScript Array to JSON
const names = ["Dee", "Fred", "Inioluwa", "Iteoluwa"]; const jsonString = JSON.stringify(names); console.log(names); // Original JS array console.log(jsonString); // JSON string
Example: JavaScript Object to JSON
const person = { name: "Damilare", age: 30, isEmployed: true, hobbies: ["Singing", "Reading", "Coding", "Helping"] }; const jsonString = JSON.stringify(person); console.log(person); // Original JS object console.log(jsonString); // JSON string
2. Convert JSON to JavaScript
Use JSON.parse() to convert a JSON string into a JavaScript object or array.
Example: JSON String to JavaScript Array
const jsonArray = `["Dee", "Fred", "Inioluwa", "Iteoluwa"]`; const jsArray = JSON.parse(jsonArray); console.log(jsonArray); // JSON string console.log(jsArray); // JS array
Example: JSON String to JavaScript Object
const jsonObject = `{ "name": "Damilare", "age": 30, "isEmployed": true, "hobbies": ["Singing", "Reading", "Coding", "Helping"] }`; const jsObject = JSON.parse(jsonObject); console.log(jsonObject); // JSON string console.log(jsObject); // JS object
Fetching and Manipulating JSON Files
JSON data can be fetched and manipulated dynamically from a server or a local file.
1. Fetching JSON Files
Use the fetch() API to request JSON data.
Example: Fetch JSON File
fetch("people.json") .then(response => response.json()) // Convert response to JS object/array .then(data => console.log(data)); // Log the JSON data
2. Iterating Through JSON Data
If the fetched JSON is an array of objects, you can use methods like .forEach() to iterate through each element.
Example: Iterating Through Fetched JSON Data
fetch("people.json") .then(response => response.json()) .then(people => { people.forEach(person => { console.log(person.name); // Access properties of each object }); });
Use Cases of JSON in Applications:
1.Configuration Files:
- JSON is used to store app settings (e.g., config.json).
2.APIs:
- REST APIs commonly return JSON as the response format.
3.Data Storage:
- Lightweight databases (e.g., Firebase, MongoDB) rely on JSON-like structures.
4.Data Interchange:
- JSON is used for communication between frontend and backend.
Reflection
What I Learned:
- How to convert JavaScript objects/arrays to JSON and vice versa.
- Using fetch() to request JSON data from an external file or API.
- Iterating through JSON data using .forEach().
Daily growth is sweet, even though it takes extra discipline.
Day 18 crushed
The above is the detailed content of My React Journey: Day 18. For more information, please follow other related articles on the PHP Chinese website!
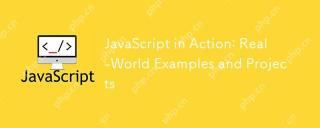
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
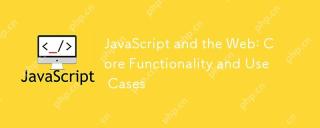
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
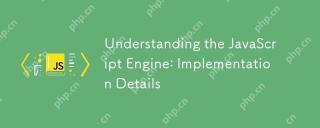
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
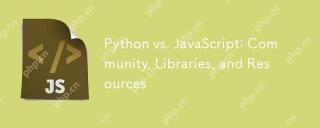
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
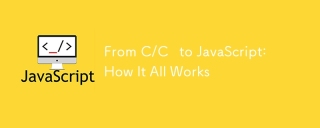
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
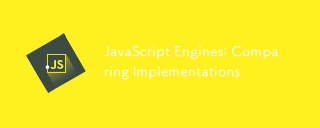
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
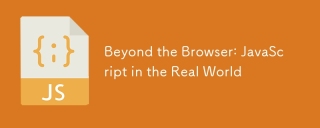
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
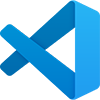
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft