Singleton Implementation with Enumerations
Singleton design patterns ensure that a class has only one instance, providing centralized access. An interesting approach to implementing Singletons in Java is through enumerations.
Consider the following enum:
public enum MySingleton { INSTANCE; }
How does this work, given that instantiation is typically performed using constructors? The answer lies in the implicit empty constructor for enum fields.
Explicit Construction
To make the instantiation process more explicit, we can define a private constructor:
public enum MySingleton { INSTANCE; private MySingleton() { System.out.println("Instance created"); } }
Instantiation
When subsequent code references the enum (such as MySingleton.INSTANCE), the constructor executes only once, establishing the singleton instance.
public static void main(String[] args) { System.out.println(MySingleton.INSTANCE); }
Output:
Instance created INSTANCE
Key Points
Enum fields are essentially instances of their enum type. They are constructed when the enum is referenced for the first time. This approach provides an elegant and type-safe means of implementing Singleton in Java.
The above is the detailed content of How Can Java Enums Be Used to Implement the Singleton Design Pattern?. For more information, please follow other related articles on the PHP Chinese website!
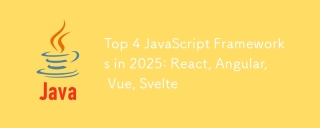
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
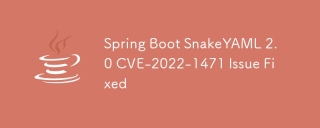
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
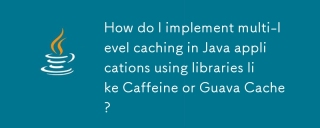
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
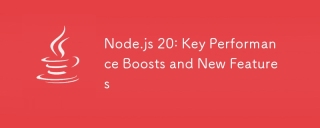
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
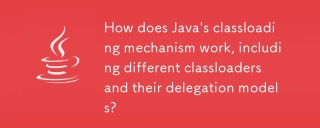
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
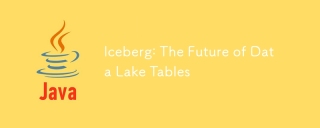
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
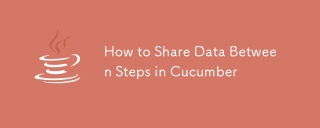
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
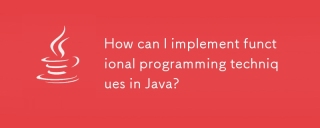
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
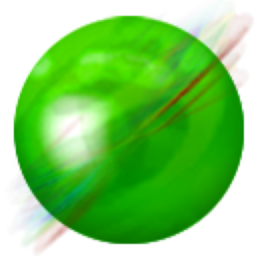
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
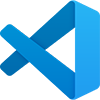
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
