


Unraveling the Enumerate() Function in Python
In Python, the enumerate() function is an enigmatic gem that adds a dash of enlightenment to your iterable explorations. What exactly does it do? Let's delve into its concept and applications.
Meaning of enumerate() in Python
At its core, enumerate() is an iterative beautifier. It introduces a counter to an iterable, transforming each element into a tuple that contains the counter and the element. This enhancement provides a clear and concise way to monitor your traversal through the iterable, offering a numerical dimension to your loop adventures.
Consider this simple example:
elements = ('foo', 'bar', 'baz') for elem in elements: print(elem)
This code will print each element of the tuple in order:
foo bar baz
However, if we invoke the enumerate() function, our loop takes on a new form:
elements = ('foo', 'bar', 'baz') for count, elem in enumerate(elements): print(count, elem)
Now, the output reveals not only the elements themselves but also their corresponding counters:
0 foo 1 bar 2 baz
Notice how each tuple unpacks into two variables: row_number (the counter) and row (the element).
Customization and Implementations
By default, enumerate() starts its counting from 0. However, you have the flexibility to specify a starting number by providing a second integer argument. For instance:
for count, elem in enumerate(elements, 42): print(count, elem)
This code will print:
42 foo 43 bar 44 baz
Python's innate enumerate() function is a finely-tuned machine, but if you're feeling adventurous, you can re-implement it using itertools.count() or a manual counting generator function:
from itertools import count def enumerate(it, start=0): return zip(count(start), it)
or
def enumerate(it, start=0): count = start for elem in it: yield (count, elem) count += 1
These custom implementations mirror Python's approach, providing a versatile tool for your looping endeavors.
The above is the detailed content of How Does Python's `enumerate()` Function Enhance Iterable Traversal?. For more information, please follow other related articles on the PHP Chinese website!
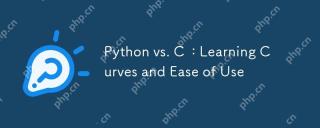
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
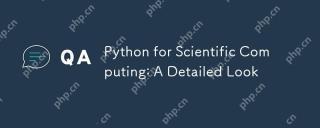
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
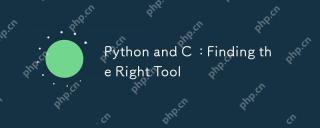
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
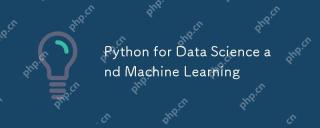
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
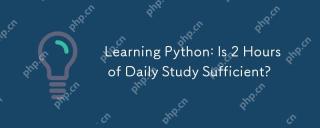
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
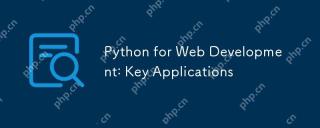
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
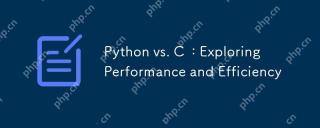
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
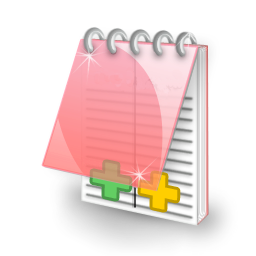
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
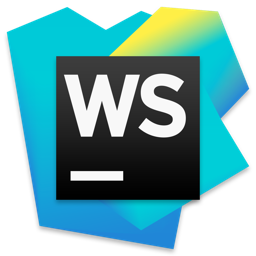
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.