Implementing SwingWorker in Java
In your previous question, you sought guidance on using SwingWorker to avoid GUI lock-ups during long-running tasks. SwingWorker provides a sophisticated mechanism for this purpose, allowing execution of such tasks on separate threads.
Understanding SwingWorker
SwingWorker is a utility class designed to handle time-consuming operations in Swing applications. Its primary purpose is to prevent the Event Dispatch Thread (EDT) from freezing, which occurs when computationally heavy tasks are executed on the EDT, blocking GUI interactions.
Implementation with your Code Snippet
To use SwingWorker with your code snippet, you can create a class extending SwingWorker, similar to the "AnswerWorker" example provided in the answer you received. This class should define a doInBackground method to execute the long-running task and a done method to perform actions once the task is complete.
Example Implementation
class MySwingWorker extends SwingWorker<integer integer> { @Override protected Integer doInBackground() throws Exception { // Execute your time-consuming task here return 42; // Replace with the result of your task } @Override protected void done() { try { // Perform actions after the task is finished using the get() method to access the result } catch (Exception e) { e.printStackTrace(); } } }</integer>
Invoking SwingWorker Using ActionListener
You can then invoke your SwingWorker using an execute method. In your case, you can attach an ActionListener to a JButton to trigger the execution:
JButton button = new JButton("Execute Task"); button.addActionListener(e -> new MySwingWorker().execute());
This will execute the SwingWorker when the button is clicked, allowing the GUI to remain responsive during the task's execution.
Conclusion
By utilizing SwingWorker effectively, you can execute time-consuming operations without compromising the responsiveness of your Swing GUI. Refer to the Java Tutorial for further information and examples on SwingWorker implementation.
The above is the detailed content of How Can SwingWorker Prevent GUI Freezes During Long-Running Java Tasks?. For more information, please follow other related articles on the PHP Chinese website!
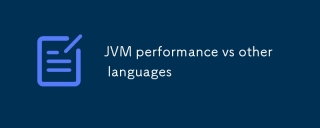
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
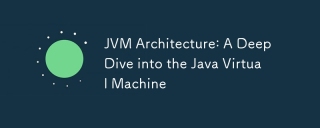
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
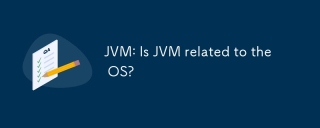
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
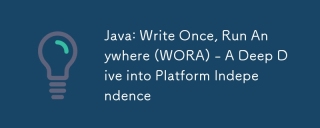
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
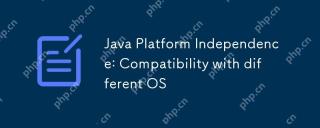
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
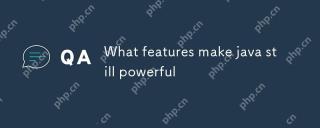
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
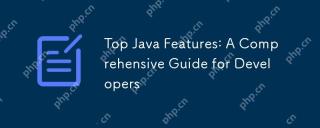
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
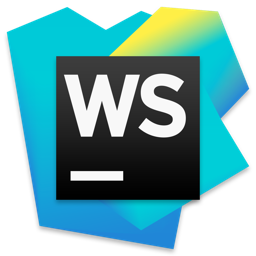
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
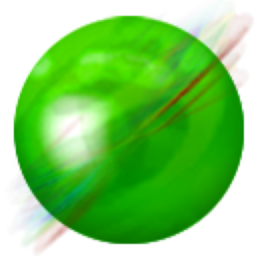
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
