


Shared Mutex with Boost
In a multithreaded environment, data access needs to be synchronized to avoid concurrent access and data corruption. Boost provides a convenient solution for this with boost::shared_mutex, allowing multiple threads to read data simultaneously while preventing writes during those reads.
Usage Overview
To use boost::shared_mutex, multiple threads can acquire read locks (boost::shared_lock) to access data without blocking other readers. When a thread needs to write, it can acquire an upgrade lock (boost::upgrade_lock). If the data is already read-locked, the upgrade lock can wait for all read locks to release before acquiring exclusive access (boost::upgrade_to_unique_lock). Alternatively, an unconditional write lock (boost::unique_lock) can be acquired to block all other threads from accessing the data.
Code Example
The following code demonstrates the usage of boost::shared_mutex:
boost::shared_mutex _access; void reader() { boost::shared_lock<:shared_mutex> lock(_access); // Read data without blocking other readers } void conditional_writer() { boost::upgrade_lock<:shared_mutex> lock(_access); // Read data without exclusive access if (condition) { boost::upgrade_to_unique_lock<:shared_mutex> uniqueLock(lock); // Write data with exclusive access } // Continue reading without exclusive access } void unconditional_writer() { boost::unique_lock<:shared_mutex> lock(_access); // Write data with exclusive access }</:shared_mutex></:shared_mutex></:shared_mutex></:shared_mutex>
Note:
- Conditional writers cannot upgrade their lock while other conditional writers hold locks.
- If all readers are conditional writers, an alternative solution is required to handle write operations.
The above is the detailed content of How Does Boost::shared_mutex Handle Concurrent Read and Write Access in Multithreaded Environments?. For more information, please follow other related articles on the PHP Chinese website!
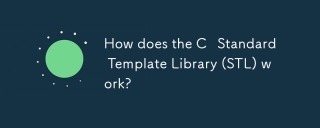
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
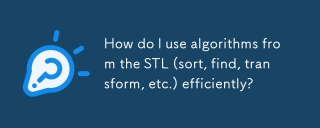
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
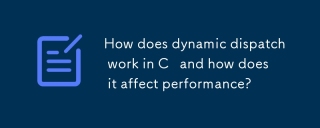
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
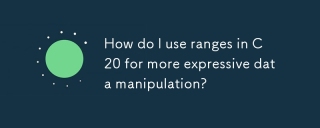
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
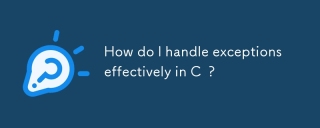
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
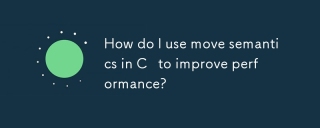
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
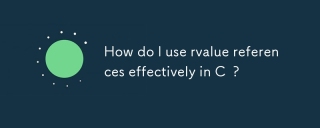
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
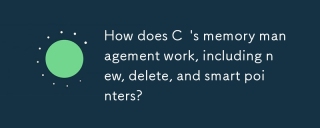
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
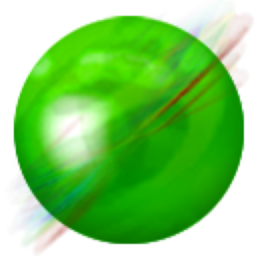
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
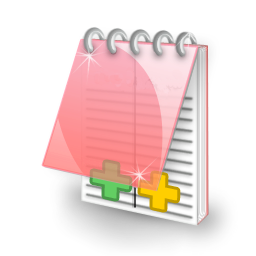
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
