Don't Let Globals Hamper Your Code: An In-Depth Discussion
While global variables can offer a quick and easy way to access data across multiple files, they can also introduce subtle dependencies and unexpected consequences. Let's delve into why using global variables in PHP can be detrimental and how to create cleaner and more maintainable code without them.
The Perils of Global Scope
The main issue with global variables is that they tightly couple different parts of your code. This can lead to unforeseen difficulties and inefficiencies when attempting to modify or reuse code.
Consider the example below, where all code in the file depends on the existence of a $config variable. Modifying this variable requires updating every line of code that accesses it, making maintenance a nightmare.
require 'SomeClass.php'; $class = new SomeClass; $class->doSomething();
In contrast, explicitly passing the config array as a parameter allows for more modular and reusable code.
require 'SomeClass.php'; $arbitraryConfigVariableName = array(...); $class = new SomeClass($arbitraryConfigVariableName); $class->doSomething();
Decoupling for Maintainability
As your codebase grows, it's crucial to decouple its components to maintain flexibility and ease of testing. Using global variables undermines this principle, creating a tangled web of dependencies that can hinder code reuse and flexibility. By passing data explicitly as parameters, you establish clear interfaces between different code blocks, making them more modular and interchangeable.
A Real-Life Example
Consider the task of accessing database information from multiple functions and classes. Instead of using global variables for $db, $config, and other database-related data, we can create separate classes and objects to manage these dependencies.
require_once 'Database.php'; require_once 'ConfigManager.php'; require_once 'Log.php'; require_once 'Foo.php'; // Database connection $db = new Database('localhost', 'user', 'pass'); // Config manager $configManager = new ConfigManager; $config = $configManager->loadConfigurationFromDatabase($db); // Logger $log = new Log($db); // Fo $foo = new Foo($config); // Conversion function $foo->conversion('foo', array('bar', 'baz'), $log);
This approach explicitly passes dependencies as parameters, improving maintainability and decoupling the code components.
Conclusion
While global variables may seem convenient, their misuse can lead to hidden dependencies and maintenance difficulties. By adopting a more object-oriented approach and passing data explicitly as parameters, you can create cleaner, more modular, and more maintainable codebases.
The above is the detailed content of Why Are Global Variables Harmful to Your PHP Code?. For more information, please follow other related articles on the PHP Chinese website!
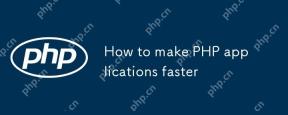
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
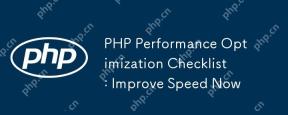
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
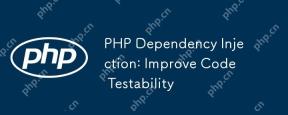
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
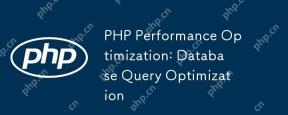
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
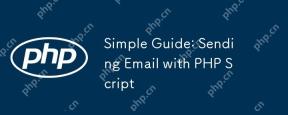
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
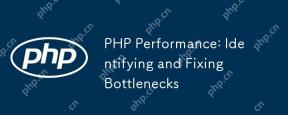
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
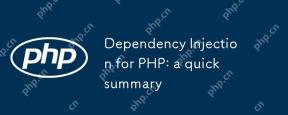
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
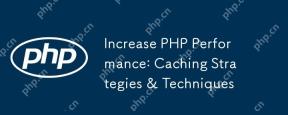
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
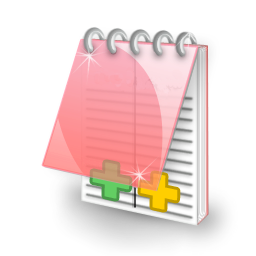
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
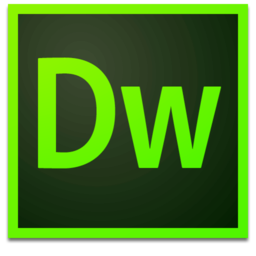
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
